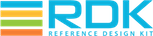 |
RDK-B
|
Go to the documentation of this file.
74 #ifndef __PLATFORM_HAL_H__
75 #define __PLATFORM_HAL_H__
107 #define UCHAR unsigned char
111 #define BOOLEAN unsigned char
119 #define UINT unsigned int
123 #define ULONG unsigned long
143 #define RETURN_ERR -1
151 #define DHCPV6_OPT_82 82 // OPTION_SOL_MAX_RT: Solicite Maximum Retry Time
152 #define DHCPV6_OPT_23 23 // OPTION_SOL_MAX_RT: Solicite Maximum Retry Time
153 #define DHCPV6_OPT_95 95 // OPTION_SOL_MAX_RT: Solicite Maximum Retry Time
154 #define DHCPV6_OPT_24 24 // OPTION_DOMAIN_LIST
155 #define DHCPV6_OPT_83 83 // OPTION_INF_MAX_RT
156 #define DHCPV6_OPT_17 17 // OPTION_VENDOR_OPTS
157 #define DHCPV6_OPT_31 31 // OPTION_SNTP_SERVERS
158 #define DHCPV6_OPT_15 15 // User Class Option
159 #define DHCPV6_OPT_16 16 // Vendor Class Option
160 #define DHCPV6_OPT_20 20 // Reconfigure Accept Option
164 #define DHCPV4_OPT_42 42 // NTP Server Addresses
165 #define DHCPV4_OPT_43 43 // Vendor Specific Information
166 #define DHCPV4_OPT_58 58 // DHCP Renewal (T1) Time
167 #define DHCPV4_OPT_59 59 // DHCP Rebinding (T2) Time
168 #define DHCPV4_OPT_60 60 // Class Identifier
169 #define DHCPV4_OPT_61 61 // Client Identifier
170 #define DHCPV4_OPT_100 100 // IEEE 1003.1 TZ String
171 #define DHCPV4_OPT_122 122 // CableLabs Client Configuration
172 #define DHCPV4_OPT_125 125 // Vendor-Identifying Vendor-Specific Information
173 #define DHCPV4_OPT_242 242 // Private Use
174 #define DHCPV4_OPT_243 243 // Private Use
175 #define DHCPV4_OPT_END 255 // DHCP Option End - used to check if option is valid
187 #ifndef PLAT_PROC_MEM_MAX_LEN
188 #define PLAT_PROC_MEM_MAX_LEN 40
729 #define LED_BUFFER_SIZE 3
745 #ifdef FEATURE_RDKB_LED_MANAGER
764 #ifdef FEATURE_RDKB_LED_MANAGER
774 int platform_hal_initLed (
char * config_file_name);
858 #ifdef FEATURE_RDKB_THERMAL_MANAGER
869 typedef struct _FAN_PLATFORM_CONFIG
872 UINT SlowSpeedThresh;
873 UINT MediumSpeedThresh;
874 UINT FastSpeedThresh;
876 UINT MonitoringDelay;
877 UINT PowerMonitoring;
878 } THERMAL_PLATFORM_CONFIG;
890 INT platform_hal_initThermal(THERMAL_PLATFORM_CONFIG* pThermalPlatformConfig);
900 INT platform_hal_LoadThermalConfig (THERMAL_PLATFORM_CONFIG* pThermalPlatformConfig);
905 FAN_ERR_MAX_OVERRIDE_SET = 2
920 INT platform_hal_setFanSpeed(
UINT fanIndex, FAN_SPEED fanSpeed, FAN_ERR* pErrReason);
932 INT platform_hal_getFanTemperature(
int* pTemp);
943 INT platform_hal_getInputCurrent(
INT *pValue);
953 INT platform_hal_getInputPower(
INT *pValue);
963 INT platform_hal_getRadioTemperature(
INT radioIndex,
INT* pValue);
int platform_hal_GetHardware(char *pValue)
int platform_hal_getRotorLock(unsigned int fanIndex)
int State
0 for Solid, 1 for Blink
Traffic_client_t Client[256]
int platform_hal_GetCPUSpeed(char *cpuSpeed)
int platform_hal_GetWebUITimeout(unsigned long *pValue)
int platform_hal_GetHardware_MemFree(char *pValue)
LED_COLOR LedColor
LED_COLOR.
int platform_hal_SetDeviceCodeImageTimeout(int seconds)
int platform_hal_getFactoryCmVariant(char *pValue)
int platform_hal_GetHardware_MemUsed(char *pValue)
int platform_hal_GetSerialNumber(char *pValue)
int Interval
In case fs State is blink then interval per second.
int platform_hal_GetFreeMemorySize(unsigned long *pulSize)
int platform_hal_SetWebAccessLevel(int userIndex, int ifIndex, unsigned long value)
int platform_hal_GetMemoryPaths(RDK_CPUS index, PPLAT_PROC_MEM_INFO *ppinfo)
struct Traffic_client * pTraffic_client_t
unsigned char platform_hal_getFanStatus(unsigned int fanIndex)
int platform_hal_getDscpClientList(WAN_INTERFACE interfaceType, pDSCP_list_t pDSCP_List)
int platform_hal_resetDscpCounts(WAN_INTERFACE interfaceType)
struct _LEDMGMT_PARAMS LEDMGMT_PARAMS
int platform_hal_GetFactoryResetCount(unsigned long *pulSize)
int platform_hal_SetWebUITimeout(unsigned long value)
int platform_hal_GetModelName(char *pValue)
int platform_hal_GetWebAccessLevel(int userIndex, int ifIndex, unsigned long *pValue)
int platform_hal_GetHardwareVersion(char *pValue)
int platform_hal_GetTotalMemorySize(unsigned long *pulSize)
int platform_hal_ClearResetCount(unsigned char bFlag)
int platform_hal_GetMACsecOperationalStatus(int ethPort, unsigned char *pFlag)
enum PSM_STATE * PPSM_STATE
int platform_hal_SetSNMPEnable(char *pValue)
struct dhcp_opt_list * next
int platform_hal_SetSSHEnable(unsigned char Flag)
int platform_hal_setFanMaxOverride(unsigned char bOverrideFlag, unsigned int fanIndex)
struct dhcp_opt_list dhcp_opt_list
int platform_hal_GetSNMPEnable(char *pValue)
unsigned int platform_hal_getRPM(unsigned int fanIndex)
int platform_hal_setDscp(WAN_INTERFACE interfaceType, TRAFFIC_CNT_COMMAND cmd, char *pDscpVals)
unsigned int platform_hal_getFanSpeed(unsigned int fanIndex)
int platform_hal_StopMACsec(int ethPort)
int platform_hal_GetDhcpv4_Options(dhcp_opt_list **req_opt_list, dhcp_opt_list **send_opt_list)
int platform_hal_getFactoryPartnerId(char *pValue)
int platform_hal_GetSoftwareVersion(char *pValue, unsigned long maxSize)
int platform_hal_StartMACsec(int ethPort, int timeoutSec)
struct DSCP_list * pDSCP_list_t
int platform_hal_GetFirmwareName(char *pValue, unsigned long maxSize)
int platform_hal_GetUsedMemorySize(unsigned long *pulSize)
int platform_hal_SetLowPowerModeState(PPSM_STATE pState)
int platform_hal_GetTelnetEnable(unsigned char *pFlag)
struct DSCP_Element * pDSCP_Element_t
int platform_hal_getTimeOffSet(char *timeOffSet)
struct DSCP_Element DSCP_Element_t
int platform_hal_getCMTSMac(char *pValue)
int platform_hal_DocsisParamsDBInit(void)
int platform_hal_GetBaseMacAddress(char *pValue)
int platform_hal_GetSSHEnable(unsigned char *pFlag)
int platform_hal_setLed(PLEDMGMT_PARAMS pValue)
int platform_hal_GetMACsecEnable(int ethPort, unsigned char *pFlag)
int platform_hal_getLed(PLEDMGMT_PARAMS pValue)
int platform_hal_setFactoryCmVariant(char *pValue)
struct DSCP_list DSCP_list_t
int platform_hal_SetMACsecEnable(int ethPort, unsigned char Flag)
struct Traffic_client Traffic_client_t
struct _LEDMGMT_PARAMS * PLEDMGMT_PARAMS
int platform_hal_SetDeviceCodeImageValid(unsigned char flag)
int platform_hal_PandMDBInit(void)
int platform_hal_GetBootloaderVersion(char *pValue, unsigned long maxSize)
int platform_hal_SetTelnetEnable(unsigned char Flag)
int platform_hal_GetDeviceConfigStatus(char *pValue)
int platform_hal_GetDhcpv6_Options(dhcp_opt_list **req_opt_list, dhcp_opt_list **send_opt_list)
int platform_hal_GetRouterRegion(char *pValue)
int platform_hal_SetSNMPOnboardRebootEnable(char *pValue)