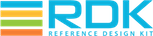 |
RDK-B
|
Go to the documentation of this file.
25 #ifndef _DHCP4_CLIENT_API_
26 #define _DHCP4_CLIENT_API_
68 #define MAX_IPV4_ADDR_LIST_NUMBER 4
int dhcp4c_get_ecm_dns_svrs(ipv4AddrList_t *pList)
int dhcp4c_get_ert_config_attempts(int *pValue)
int dhcp4c_get_ecm_ip_addr(unsigned int *pValue)
int dhcp4c_get_ert_ifname(char *pName)
int dhcp4c_get_ecm_mask(unsigned int *pValue)
int dhcp4c_get_ert_mask(unsigned int *pValue)
@ DHCPC_CMD_CONFIG_ATTEMPTS
int dhcp4c_get_ert_fsm_state(int *pValue)
int dhcp4c_get_ecm_ifname(char *pName)
int dhcp4c_get_emta_remain_renew_time(unsigned int *pValue)
int dhcp4c_get_ert_remain_rebind_time(unsigned int *pValue)
int dhcp4c_get_emta_remain_rebind_time(unsigned int *pValue)
int dhcp4c_get_ecm_remain_rebind_time(unsigned int *pValue)
int dhcp4c_get_emta_remain_lease_time(unsigned int *pValue)
int dhcp4c_get_ert_lease_time(unsigned int *pValue)
int dhcp4c_get_ecm_config_attempts(int *pValue)
int dhcp4c_get_ecm_dhcp_svr(unsigned int *pValue)
int dhcp4c_get_ecm_remain_renew_time(unsigned int *pValue)
int dhcp4c_get_ecm_lease_time(unsigned int *pValue)
int dhcp4c_get_ert_ip_addr(unsigned int *pValue)
int dhcp4c_get_ert_dns_svrs(ipv4AddrList_t *pList)
#define MAX_IPV4_ADDR_LIST_NUMBER
@ DHCPC_CMD_RENEW_TIME_REMAIN
int dhcp4c_get_ecm_remain_lease_time(unsigned int *pValue)
int dhcp4c_get_ecm_fsm_state(int *pValue)
int dhcp4c_get_ert_dhcp_svr(unsigned int *pValue)
int dhcp4c_get_ert_remain_lease_time(unsigned int *pValue)
@ DHCPC_CMD_LEASE_TIME_REMAIN
int dhcp4c_get_ert_remain_renew_time(unsigned int *pValue)
int dhcp4c_get_ecm_gw(unsigned int *pValue)
int dhcp4c_get_ert_gw(unsigned int *pValue)
@ DHCPC_CMD_REBIND_TIME_REMAIN