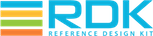 |
RDK-B
|
Go to the documentation of this file.
31 #define XDSL_MAX_LINES 1
133 char FirmwareVersion[64];
135 char StandardsSupported[256];
136 unsigned char XTSE[17];
137 char StandardUsed[64];
138 unsigned char XTSUsed[17];
140 char AllowedProfiles[256];
141 char CurrentProfile[64];
146 char UPBOKLERPb[256];
174 char VirtualNoisePSDds[97];
175 char VirtualNoisePSDus[49];
187 char XTURVendor[8+1];
188 char XTURCountry[4+1];
191 char XTUCVendor[8+1];
192 char XTUCCountry[4+1];
354 char DiagnosticsState[64];
373 char HLINpsds[61430];
374 char HLINpsus[61430];
387 char BITSpsds[61430];
388 char BITSpsus[61430];
393 char DiagnosticsState[64];
405 char DiagnosticsState[64];
415 char DiagnosticsState[64];
417 char CapacitySignalPSD[1024];
418 char CapacityNoisePSD[1024];
420 char LoopTermination[24];
422 char LoopTopology[256];
423 char AttenuationCharacteristics[61430];
enum _DslLinkStatus_t DslLinkStatus_t
_DslPowerManagementState_t
unsigned int X_RDK_LinkRetrain
DslChannelError_t StatsLastShowtime
DslLineCurrentDay_t StatsCurrentDay
unsigned int X_RDK_InitErrors
bool CapacityEstimateEnabling
bool ExtendedBandwidthOperation
unsigned int XTUCCRCErrors
@ ADSL_DIAG_STATE_Complete
unsigned long long BytesSent
int xdsl_hal_dslGetXRdk_Nlm(DslXRdkNlm_t *nlmInfo)
DslLineEncoding_t LineEncoding
int xdsl_hal_getTotalNoOfLines(void)
unsigned int UERScaleFactor
@ LINK_ENCAP_G_993_2_Annex_K_PTM
unsigned int LastStateTransmittedUpstream
unsigned int XTUCHECErrors
unsigned int DiscardPacketsReceived
unsigned int XTURCRCErrors
unsigned int QLNMaxMeasurementDuration
int xdsl_hal_dslGetLineInfo(int lineNo, DslLineInfo_t *lineInfo)
int xdsl_hal_dslDiagnosticTestStart(int lineNo, DslDiagTestType_t type)
unsigned int CapacityTargetMargin
unsigned int X_RDK_LinkRetrain
DslLineError_t StatsTotal
unsigned int CurrentDayStart
unsigned int X_RDK_LinkRetrain
int xdsl_hal_registerDslLinkStatusCallback(dsl_link_status_callback link_status_cb)
unsigned int SeverelyErroredSecs
enum _DslProfile_t DslProfile_t
int DownstreamNoiseMargin
enum _DslLineEncoding_t DslLineEncoding_t
unsigned int UpstreamMaxBitRate
DslPowerManagementState_t PowerManagementState
@ LINK_ENCAP_G_994_1_Auto
unsigned int X_RDK_InitTimeouts
unsigned long long BytesSent
unsigned int XTURCRCErrors
@ ADSL_DIAG_STATE_Error_Other
int xdsl_hal_dslGetDiagnosticSeltuer(int lineNo, DslDiagSeltuer_t *DiagSeltuer)
int xdsl_hal_dslGetDiagnosticSeltqln(int lineNo, DslDiagSeltqln_t *DiagSeltqln)
DslLineError_t StatsShowtime
unsigned int XTURHECErrors
unsigned int QLNGroupSize
unsigned int XTURHECErrors
unsigned long long PacketsSent
DslDiagSeltp_t stDiagSELTP
unsigned int X_RDK_InitErrors
int xdsl_hal_getTotalNoofChannels(int lineNo)
int xdsl_hal_dslGetLineEnable(int lineNo, unsigned char *enable)
unsigned int XTUCCRCErrors
unsigned int XTURCRCErrors
enum _DslLineTrainingStatus_t DslLineTrainingStatus_t
DslChannelQuarterHour_t StatsQuarterHour
int xdsl_hal_dslGetChannelStats(int lineNo, int channelNo, DslChannelStats_t *channelStats)
unsigned int CapacityEstimate
int DownstreamAttenuation
unsigned int XTUCCRCErrors
unsigned int UERGroupSize
enum _DslModType_t DslModType_t
unsigned int XTUCFECErrors
unsigned int XTUCFECErrors
unsigned int XTUCHECErrors
DslDiagSeltqln_t stDiagSELTQLN
unsigned long long PacketsSent
@ ADSL_DIAG_STATE_Canceled
unsigned int DownstreamCurrRate
unsigned int SeverelyErroredSecs
DslLinkStatus_t LinkStatus
unsigned int LastShowtimeStart
unsigned int XTURHECErrors
unsigned int SuccessFailureCause
@ LINK_ENCAP_G_992_3_Annex_K_ATM
bool ExtendedBandwidthOperation
unsigned int UERMaxMeasurementDuration
unsigned int X_RDK_InitTimeouts
unsigned int DownstreamMaxBitRate
int xdsl_hal_dslGetDiagnosticAdslLineTest(int lineNo, DslDiagAdslLineTest_t *AdslLineTest)
unsigned int XTURFECErrors
DslChannelError_t StatsTotal
unsigned int ShowtimeStart
DslDiagAdslLineTest_t stDiagADSLLineTest
unsigned int X_RDK_LinkRetrain
unsigned int X_RDK_ErroredSecs
int(* dsl_link_status_callback)(char *ifname, DslLinkStatus_t dsl_status)
int xdsl_hal_dslSetLineEnable(int lineNo, unsigned char enable)
@ LINK_ENCAP_G_993_2_Annex_K_ATM
int xdsl_hal_dslSetLineEnableDataGathering(int lineNo, unsigned char enable)
enum _IfStatus_t IfStatus_t
unsigned int DiscardPacketsSent
unsigned int UpstreamCurrRate
unsigned long long BytesReceived
@ ADSL_DIAG_STATE_Error_Internal
unsigned int LastShowtimeStart
unsigned int XTUCHECErrors
unsigned int ACTSNRMODEds
unsigned int CurrentDayStart
unsigned int ActualInterleavingDelay
unsigned int SeverelyErroredSecs
unsigned int DiscardPacketsReceived
unsigned int INMINPEQMODEds
unsigned int XTUCFECErrors
unsigned int ErrorsReceived
unsigned int ErrorsReceived
DslLineQuarterHour_t StatsQuarterHour
int xdsl_hal_dslGetChannelInfo(int lineNo, int channelNo, DslChannel_t *channelInfo)
DslChannelCurrentDay_t StatsCurrentDay
unsigned int XTURFECErrors
@ LINK_ENCAP_G_992_3_Annex_K_PTM
DslLineError_t StatsLastShowtime
unsigned int X_RDK_SeverelyErroredSecs
unsigned long long BytesReceived
unsigned int DiscardPacketsSent
unsigned long long PacketsReceived
@ ADSL_DIAG_STATE_Requested
unsigned int QuarterHourStart
DslChannelError_t StatsShowtime
int xdsl_hal_dslGetLineStats(int lineNo, DslLineStats_t *lineStats)
unsigned long long PacketsReceived
unsigned int X_RDK_SuccessfulRetrains
int xdsl_hal_dslGetDiagnosticSeltp(int lineNo, DslDiagSeltp_t *DiagSeltp)
enum _DslPowerManagementState_t DslPowerManagementState_t
unsigned int ShowtimeStart
unsigned int XTURFECErrors
DslDiagSeltuer_t stDiagSELTUER
unsigned int LastStateTransmittedDownstream
unsigned int QuarterHourStart