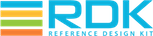 |
RDK-B
|
Go to the documentation of this file.
80 #include <netinet/in.h>
105 #define ULONG unsigned long
113 #define UCHAR unsigned char
117 #define BOOLEAN UCHAR
141 #define RETURN_ERR -1
144 #ifndef IPV4_ADDRESS_SIZE
145 #define IPV4_ADDRESS_SIZE 4
148 #ifndef MTA_HAL_SHORT_VALUE_LEN
149 #define MTA_HAL_SHORT_VALUE_LEN 16
152 #ifndef MTA_HAL_LONG_VALUE_LEN
153 #define MTA_HAL_LONG_VALUE_LEN 64
156 #ifndef ANSC_IPV4_ADDRESS
162 #define ANSC_IPV4_ADDRESS \
165 unsigned char Dot[IPV4_ADDRESS_SIZE]; \
205 #define DECT_MAX_HANDSETS 5
214 ULONG RegisterDectHandset;
215 ULONG DeregisterDectHandset;
216 char HardwareVersion[64];
218 char SoftwareVersion[64];
228 char LastActiveTime[64];
229 char HandsetName[64];
230 char HandsetFirmware[64];
231 char OperatingTN[64];
232 char SupportedTN[64];
263 CHAR IPV6Address[INET6_ADDRSTRLEN];
267 CHAR Gateway[INET6_ADDRSTRLEN];
271 CHAR PrimaryDNS[INET6_ADDRSTRLEN];
272 CHAR SecondaryDNS[INET6_ADDRSTRLEN];
279 CHAR PrimaryDHCPv6Server[INET6_ADDRSTRLEN];
280 CHAR SecondaryDHCPv6Server[INET6_ADDRSTRLEN];
434 CHAR ChargerFirmwareRevision[32];
438 #define MTA_LINENUMBER 8
1320 #define MTA_DHCPOPTION122SUBOPTION1_MAX 4
1321 #define MTA_DHCPOPTION122SUBOPTION2_MAX 4
1322 #define MTA_DHCPOPTION122CCCV6DSSID1_MAX 32
1323 #define MTA_DHCPOPTION122CCCV6DSSID2_MAX 32
int mta_hal_BatteryGetTotalCapacity(unsigned long *Val)
int mta_hal_GetHandsets(unsigned long *pulCount, PMTAMGMT_MTA_HANDSETS_INFO *ppHandsets)
unsigned long LeaseTimeRemaining
unsigned long NomGrantInterval
int(* mta_hal_getLineRegisterStatus_callback)(MTAMGMT_MTA_STATUS *output_status_array, int array_size)
struct _MTAMGMT_MTA_DSXLOG * PMTAMGMT_MTA_DSXLOG
int mta_hal_getLineRegisterStatus(MTAMGMT_MTA_STATUS *output_status_array, int array_size)
int mta_hal_devResetNow(unsigned char bResetValue)
int mta_hal_BatteryGetInfo(PMTAMGMT_MTA_BATTERY_INFO pInfo)
struct _MTAMGMT_MTA_SERVICE_FLOW * PMTAMGMT_MTA_SERVICE_FLOW
MTAMGMT_MTA_PROVISION_STATUS
int mta_hal_BatteryGetNumberofCycles(unsigned long *Val)
int mta_hal_GetDHCPInfo(PMTAMGMT_MTA_DHCP_INFO pInfo)
int mta_hal_getConfigFileStatus(MTAMGMT_MTA_STATUS *poutput_status)
int mta_hal_GetCalls(unsigned long InstanceNumber, unsigned long *Count, PMTAMGMT_MTA_CALLS *ppCfg)
struct _MTAMGMT_MTA_CALLS * PMTAMGMT_MTA_CALLS
unsigned long NomPollInterval
int mta_hal_SetCallSignallingLogEnable(unsigned char Bool)
int mta_hal_GetDect(PMTAMGMT_MTA_DECT pDect)
#define MTA_DHCPOPTION122CCCV6DSSID2_MAX
unsigned char JitterBufferAdaptive
struct _MTAMGMT_PROVISIONING_PARAMS MTAMGMT_PROVISIONING_PARAMS
struct _MTAMGMT_MTA_DHCP_INFO MTAMGMT_MTA_DHCP_INFO
unsigned long MaxTrafficRate
#define MTA_HAL_SHORT_VALUE_LEN
unsigned char DefaultFlow
unsigned long MinReservedPkt
struct _MTAMGMT_MTA_MTALOG_FULL MTAMGMT_MTA_MTALOG_FULL
int mta_hal_GetDSXLogEnable(unsigned char *pBool)
struct _MTAMGMT_MTA_DHCPv6_INFO * PMTAMGMT_MTA_DHCPv6_INFO
int mta_hal_BatteryGetInstalled(unsigned char *Val)
int mta_hal_BatteryGetRemainingCharge(unsigned long *Val)
unsigned long MinReservedRate
char DhcpOption2171CccV6DssID1[32+1]
struct _MTAMGMT_MTA_DECT MTAMGMT_MTA_DECT
unsigned long LeaseTimeRemaining
int mta_hal_GetDHCPV6Info(PMTAMGMT_MTA_DHCPv6_INFO pInfo)
struct _MTAMGMT_MTA_BATTERY_INFO * PMTAMGMT_MTA_BATTERY_INFO
int mta_hal_SetDSXLogEnable(unsigned char Bool)
int mta_hal_BatteryGetRemainingTime(unsigned long *Val)
unsigned long UnsolicitGrantSize
unsigned long CallsUpdateTime
char DhcpOption122Suboption1[4+1]
int mta_hal_BatteryGetCondition(char *Val, unsigned long *len)
struct _MTAMGMT_MTA_CALLS MTAMGMT_MTA_CALLS
int mta_hal_GetMtaLog(unsigned long *Count, PMTAMGMT_MTA_MTALOG_FULL *ppCfg)
int mta_hal_BatteryGetActualCapacity(unsigned long *Val)
unsigned long CallDuration
int mta_hal_getMtaProvisioningStatus(MTAMGMT_MTA_PROVISION_STATUS *provisionStatus)
int mta_hal_GetCALLP(unsigned long LineNumber, PMTAMGMT_MTA_CALLP pCallp)
int DhcpOption2171CccV6DssID2Len
unsigned long OverCurrentFault
struct _MTAMGMT_MTA_DSXLOG MTAMGMT_MTA_DSXLOG
unsigned long InstanceNumber
struct _MTAMGMT_MTA_HANDSETS_INFO * PMTAMGMT_MTA_HANDSETS_INFO
int mta_hal_GetDectPIN(char *pPINString)
struct _MTAMGMT_MTA_LINETABLE_INFO * PMTAMGMT_MTA_LINETABLE_INFO
struct _MTAMGMT_MTA_CALLP * PMTAMGMT_MTA_CALLP
unsigned long MaxTrafficBurst
int mta_hal_DectGetRegistrationMode(unsigned char *pBool)
unsigned long InstanceNumber
#define MTA_DHCPOPTION122CCCV6DSSID1_MAX
int mta_hal_start_provisioning(PMTAMGMT_MTA_PROVISIONING_PARAMS pParameters)
int DhcpOption2171CccV6DssID1Len
struct _MTAMGMT_MTA_BATTERY_INFO MTAMGMT_MTA_BATTERY_INFO
struct _MTAMGMT_MTA_SERVICE_FLOW MTAMGMT_MTA_SERVICE_FLOW
#define MTA_DHCPOPTION122SUBOPTION2_MAX
struct _MTAMGMT_MTA_DHCP_INFO * PMTAMGMT_MTA_DHCP_INFO
int mta_hal_DectSetRegistrationMode(unsigned char bBool)
struct _MTAMGMT_MTA_LINETABLE_INFO MTAMGMT_MTA_LINETABLE_INFO
unsigned long ScheduleType
struct _MTAMGMT_MTA_CALLP MTAMGMT_MTA_CALLP
int mta_hal_Get_MTAResetCount(unsigned long *resetcnt)
unsigned long mta_hal_LineTableGetNumberOfEntries(void)
PMTAMGMT_MTA_CALLS pCalls
#define MTA_DHCPOPTION122SUBOPTION1_MAX
unsigned char RemoteJitterBufferAdaptive
int mta_hal_BatteryGetPowerStatus(char *Val, unsigned long *len)
int mta_hal_DectGetEnable(unsigned char *pBool)
int mta_hal_GetCallSignallingLogEnable(unsigned char *pBool)
int mta_hal_GetServiceFlow(unsigned long *Count, PMTAMGMT_MTA_SERVICE_FLOW *ppCfg)
struct _MTAMGMT_MTA_DECT * PMTAMGMT_MTA_DECT
int mta_hal_getDhcpStatus(MTAMGMT_MTA_STATUS *output_pIpv4status, MTAMGMT_MTA_STATUS *output_pIpv6status)
#define ANSC_IPV4_ADDRESS
int mta_hal_DectSetEnable(unsigned char bBool)
int mta_hal_TriggerDiagnostics(unsigned long Index)
int mta_hal_getMtaOperationalStatus(MTAMGMT_MTA_STATUS *operationalStatus)
int mta_hal_SetDectPIN(char *pPINString)
struct _MTAMGMT_MTA_DHCPv6_INFO MTAMGMT_MTA_DHCPv6_INFO
char DhcpOption2171CccV6DssID2[32+1]
int mta_hal_BatteryGetLife(char *Val, unsigned long *len)
unsigned long CallsNumber
int mta_hal_GetDSXLogs(unsigned long *Count, PMTAMGMT_MTA_DSXLOG *ppDSXLog)
int mta_hal_DectDeregisterDectHandset(unsigned long uValue)
unsigned long TolGrantJitter
int mta_hal_BatteryGetStatus(char *Val, unsigned long *len)
int mta_hal_Get_LineResetCount(unsigned long *resetcnt)
char DhcpOption122Suboption2[4+1]
int mta_hal_ClearDSXLog(unsigned char Bool)
unsigned long NumberOfPackets
void mta_hal_LineRegisterStatus_callback_register(mta_hal_getLineRegisterStatus_callback callback_proc)
int mta_hal_ClearCallSignallingLog(unsigned char Bool)
struct _MTAMGMT_MTA_MTALOG_FULL * PMTAMGMT_MTA_MTALOG_FULL
struct _MTAMGMT_MTA_HANDSETS_INFO MTAMGMT_MTA_HANDSETS_INFO
int mta_hal_ClearCalls(unsigned long InstanceNumber)
int mta_hal_LineTableGetEntry(unsigned long Index, PMTAMGMT_MTA_LINETABLE_INFO pEntry)
int mta_hal_BatteryGetPowerSavingModeStatus(unsigned long *pValue)
struct _MTAMGMT_PROVISIONING_PARAMS * PMTAMGMT_MTA_PROVISIONING_PARAMS