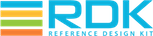 |
RDK-B
|
Go to the documentation of this file.
58 #ifndef __VLAN_HAL_H__
59 #define __VLAN_HAL_H__
66 #define ULONG unsigned long
70 #define BOOL unsigned char
78 #define UCHAR unsigned char
86 #define UINT unsigned int
106 #define RETURN_ERR -1
128 #define VLAN_HAL_MAJOR_VERSION 1
129 #define VLAN_HAL_MINOR_VERSION 0
130 #define VLAN_HAL_MAINTENANCE_VERSION 1
133 #define VLAN_HAL_MAX_VLANGROUP_TEXT_LENGTH 32
134 #define VLAN_HAL_MAX_VLANID_TEXT_LENGTH 32
135 #define VLAN_HAL_MAX_INTERFACE_NAME_TEXT_LENGTH 32
137 #define VLAN_HAL_MAX_LINE_BUFFER_LENGTH 120
int _is_this_interface_available_in_linux_bridge(char *if_name, char *vlanID)
int vlan_hal_addInterface(const char *groupName, const char *ifName, const char *vlanID)
int vlan_hal_delInterface(const char *groupName, const char *ifName, const char *vlanID)
int vlan_hal_printAllGroup()
int vlan_hal_printGroup(const char *groupName)
#define VLAN_HAL_MAX_VLANID_TEXT_LENGTH
#define VLAN_HAL_MAX_VLANGROUP_TEXT_LENGTH
struct _vlan_vlanidconfiguration * nextlink
void _get_shell_outputbuffer(char *cmd, char *out, int len)
int vlan_hal_addGroup(const char *groupName, const char *default_vlanID)
int vlan_hal_delGroup(const char *groupName)
int _is_this_interface_available_in_given_linux_bridge(char *if_name, char *br_name, char *vlanID)
int _is_this_group_available_in_linux_bridge(char *br_name)
struct _vlan_vlanidconfiguration vlan_vlanidconfiguration_t
int get_vlanId_for_GroupName(const char *groupName, char *vlanID)
int insert_VLAN_ConfigEntry(char *groupName, char *vlanID)
int delete_VLAN_ConfigEntry(char *groupName)
int print_all_vlanId_Configuration(void)
int vlan_hal_delete_all_Interfaces(const char *groupName)