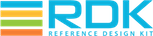 |
RDK-B
|
Go to the documentation of this file.
68 #ifndef __DPOE_HAL_H__
69 #define __DPOE_HAL_H__
94 #define UCHAR unsigned char
98 #define BOOLEAN unsigned char
102 #define USHORT unsigned short
110 #define UINT8 unsigned char
114 #define ULONG unsigned long
118 #define ULONGLONG unsigned long long
134 #define RETURN_ERR -1
137 #define DPOE_HAL_MAJOR_VERSION 1
138 #define DPOE_HAL_MINOR_VERSION 0
139 #define DPOE_HAL_MAINTENANCE_VERSION 1
struct dpoe_traffic_stats dpoe_traffic_stats_t
int dpoe_hal_Get_eponResetCount(unsigned long *resetcnt)
unsigned long long port_RxFrame64
unsigned long long port_RxFrame128_255
unsigned long info_bootCrc32
unsigned short manufacturer_DateYear
struct dpoe_epon_chip_info dpoe_epon_chip_info_t
unsigned long long port_TxFrame256_511
unsigned char capabilities_UpQueueIncrement
unsigned long long port_TxUnicastFrames
unsigned short port_OpticalMonTxPower
unsigned long long port_RxFrameTooShort
struct dpoe_device_sys_descr_info dpoe_device_sys_descr_info_t
unsigned long long port_TxFrame65_127
unsigned long long port_TxFrame128_255
struct dpoe_link_oam_frame_rate dpoe_link_oam_frame_rate_t
int dpoe_getNumberOfS1Interfaces(unsigned long *pNumS1Interfaces)
struct dpoe_mac_address dpoe_mac_address_t
unsigned short links_downstreamonly
int dpoe_setClearOnuLinkStatistics(void)
unsigned short capabilities_TotalPacketBuffer
int dpoe_getDynamicMacAddressAgeLimit(unsigned short *pAgeLimit)
dpoe_traffic_stats_t link_TrafficStats
struct dpoe_link_mac_address dpoe_link_mac_address_t
int dpoe_getDeviceSysDescrInfo(dpoe_device_sys_descr_info_t *pDeviceSysDescrInfo)
unsigned long long port_TxFrame512_1023
int dpoe_OnuLinkStatisticsGetEntryCount(unsigned short *pNumEntry)
unsigned char capabilities_UpstreamQueues
unsigned char info_HardwareVersion[32]
unsigned long long port_RxUnicastFrames
dpoe_manufacturer_date_t manufacturer_Date
unsigned short port_OpticalMonTxBiasCurrent
unsigned short info_bootVersion
unsigned short capabilities_DnPacketBuffer
int dpoe_getEponChipInfo(dpoe_epon_chip_info_t *pEponChipInfo)
unsigned long long port_TxFrame_1519_Plus
unsigned short info_JedecId
int dpoe_hal_Get_ErouterResetCount(unsigned long *resetcnt)
unsigned long long port_RxFrame65_127
unsigned long info_appCrc32
int dpoe_getEponMode(unsigned short *pMode)
struct dpoe_link_encrypt_mode dpoe_link_encrypt_mode_t
unsigned long info_ChipVersion
int dpoe_setResetOnu(void)
unsigned char info_VendorName[32]
unsigned char link_ForwardingState
int dpoe_OamFrameRateGetEntryCount(unsigned short *pNumEntry)
unsigned short info_appVersion
unsigned char macAddress[6]
int dpoe_getOamFrameRate(dpoe_link_oam_frame_rate_t linkOamFrameRate[], unsigned short numEntries)
unsigned char capabilities_UpQueuesMaxPerLink
unsigned long long port_RxFrame1519_Plus
struct dpoe_manufacturer_date dpoe_manufacturer_date_t
unsigned long long port_TxFrame_1024_1518
unsigned long long port_BytesDropped
unsigned long long port_RxFrame1024_1518
int dpoe_hal_Reboot_Ready(unsigned long *pValue)
int dpoe_getNumberOfNetworkPorts(unsigned long *pNumPorts)
unsigned char link_MinRate
int dpoe_getDynamicMacTable(dpoe_link_mac_address_t linkDynamicMacTable[], unsigned short numEntries)
int dpoe_getOnuId(dpoe_mac_address_t *pOnuId)
int dpoe_getOnuLinkStatistics(dpoe_link_traffic_stats_t onuLinkTrafficStats[], unsigned short numEntries)
struct dpoe_firmware_info dpoe_firmware_info_t
unsigned char manufacturer_DateMonth
int dpoe_getManufacturerInfo(dpoe_manufacturer_t *pManufacturerInfo)
unsigned char capabilities_DownstreamQueues
unsigned short link_EncryptExpiryTime
unsigned long long port_FramesDropped
unsigned short links_bidirectional
int dpoe_getMacLearningAggregateLimit(unsigned short *pAggregrateLimit)
struct dpoe_onu_packet_buffer_capabilities dpoe_onu_packet_buffer_capabilities_t
struct dpoe_link_forwarding_state dpoe_link_forwarding_state_t
unsigned char capabilities_DnQueuesMaxPerPort
unsigned long long port_RxFrame512_1023
int dpoe_hal_LocalResetCount(unsigned long *resetcnt)
struct dpoe_onu_max_logical_links dpoe_onu_max_logical_links_t
unsigned char manufacturer_OrganizationName[255]
unsigned long long port_RxFrame256_511
unsigned char manufacturer_DateDay
struct dpoe_link_encrypt_expiry_time dpoe_link_encrypt_expiry_time_t
struct dpoe_manufacturer dpoe_manufacturer_t
unsigned short port_OpticalMonVcc
int dpoe_getOnuPacketBufferCapabilities(dpoe_onu_packet_buffer_capabilities_t *pCapabilities)
unsigned long long port_TxFrame64
unsigned short dpoe_getMaxLogicalLinks(dpoe_onu_max_logical_links_t *pMaxLogicalLinks)
unsigned short capabilities_UpPacketBuffer
int dpoe_getDynamicMacLearningTableSize(unsigned short *pNumEntries)
unsigned char manufacturer_Info[255]
int dpoe_LlidForwardingStateGetEntryCount(unsigned short *pNumEntry)
int dpoe_getFirmwareInfo(dpoe_firmware_info_t *pFirmwareInfo)
unsigned char info_ModelNumber[32]
unsigned short port_OpticalMonRxPower
unsigned char link_MaxRate
unsigned short numEntries
dpoe_mac_address_t * pMacAddress
struct dpoe_link_traffic_stats dpoe_link_traffic_stats_t
unsigned long info_ChipModel
unsigned char capabilities_DnQueueIncrement
int dpoe_getStaticMacTable(dpoe_link_mac_address_t linkStaticMacTable[], unsigned short numEntries)
unsigned char link_EncryptMode
int dpoe_getLlidForwardingState(dpoe_link_forwarding_state_t linkForwardingState[], unsigned short numEntries)