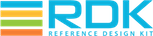 |
RDK-B
|
Go to the documentation of this file.
41 #define RETURN_ERROR 1
88 char queueStatus[256];
89 char queueInterface[256];
93 char dropAlgorithm[256];
94 char schedulerAlgorithm[256];
106 char L2DeviceType[32];
115 char brIPv6Prefix[128];
116 char ruleIPv4Prefix[128];
117 char ruleIPv6Prefix[128];
120 char pdIPv6Prefix[128];
struct _WAN_MAPT_CFG WAN_MAPT_CFG
unsigned long LowClassMinThreshold
struct _WAN_QOS_QUEUE WAN_QOS_QUEUE
int wan_hal_SetQoSConfiguration(PWAN_QOS_QUEUE pQueue, unsigned int QueueNumberOfEntries, const char *baseifname, const char *wanifname)
unsigned long InstanceNumber
struct _WAN_IPV6_CFG * PWAN_IPV6_CFG
struct _SELFHEAL_CONFIG SELFHEAL_CONFIG
struct _WAN_IPV4_CFG WAN_IPV4_CFG
int wan_hal_SetWanConnectionEnable(unsigned int enable)
struct _WAN_MAPT_CFG * PWAN_MAPT_CFG
int wan_hal_EnableMapt(PWAN_MAPT_CFG pMAPTCfg)
int wan_hal_DisableMapt(const char *ifName)
int wan_hal_UnConfigureIpv4(PWAN_IPV4_CFG pWanIpv4Cfg)
unsigned long shapingBurstSize
unsigned long HighClassMinThreshold
int wan_hal_GetWanOEUpstreamCurrRate(unsigned int *pValue)
int wan_hal_GetWanOEDownstreamCurrRate(unsigned int *pValue)
unsigned long HighClassMaxThreshold
struct _WAN_IPV4_CFG * PWAN_IPV4_CFG
unsigned int rebootStatus
struct _SELFHEAL_CONFIG * PSELFHEAL_CONFIG
int wan_hal_ConfigureIpv4(PWAN_IPV4_CFG pWanIpv4Cfg)
struct _WAN_IPV6_CFG WAN_IPV6_CFG
unsigned long queuePrecedence
unsigned char queueEnable
int wan_hal_UnConfigureIpv6(PWAN_IPV6_CFG pWanIpv6Cfg)
int wan_hal_SetWanmode(t_eWanMode mode)
int wan_hal_enableWanOEMode(const unsigned char enable)
int wan_hal_SetSelfHealConfig(PSELFHEAL_CONFIG pSelfHealConfig)
unsigned long REDThreshold
int wan_hal_ConfigureIpv6(PWAN_IPV6_CFG pWanIpv6Cfg)
struct _WAN_QOS_QUEUE * PWAN_QOS_QUEUE
int wan_hal_getAuthInfo(char *authInfo)
unsigned long LowClassMaxThreshold
unsigned long queueWeight