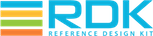 |
RDK-B
|
Go to the documentation of this file.
71 #ifndef __CCSP_HAL_ETHSW_H__
72 #define __CCSP_HAL_ETHSW_H__
96 #define ULONG unsigned long
100 #define ULLONG unsigned long long
108 #define UCHAR unsigned char
112 #define BOOLEAN UCHAR
120 #define UINT unsigned int
140 #define RETURN_ERR -1
143 #ifndef ETHWAN_DEF_INTF_NUM
149 #if defined (ETH_6_PORTS)
150 #define ETHWAN_DEF_INTF_NUM 5
151 #elif defined (ETH_4_PORTS)
152 #define ETHWAN_DEF_INTF_NUM 3
155 #define ETHWAN_DEF_INTF_NUM 0
159 #ifndef ETHWAN_INTERFACE_NAME_MAX_LENGTH
164 #define ETHWAN_INTERFACE_NAME_MAX_LENGTH 32
543 #ifdef FEATURE_RDKB_WAN_MANAGER
544 #ifdef FEATURE_RDKB_AUTO_PORT_SWITCH
545 int CcspHalExtSw_ethPortConfigure(
char *ifname,
BOOLEAN WanMode);
546 #endif //FEATURE_RDKB_AUTO_PORT_SWITCH
586 #ifdef FEATURE_RDKB_AUTO_PORT_SWITCH
597 BOOLEAN CcspHalExtSw_getCurrentWanHWConf();
706 unsigned char * Interface,
@ CCSP_HAL_ETHSW_EthPort1
int CcspHalEthSwSetPortAdminStatus(CCSP_HAL_ETHSW_PORT PortId, CCSP_HAL_ETHSW_ADMIN_STATUS AdminStatus)
int CcspHalExtSw_setEthWanPort(unsigned int Port)
unsigned long ErrorsReceived
unsigned long BroadcastPacketsSent
void CcspHalExtSw_ethAssociatedDevice_callback_register(CcspHalExtSw_ethAssociatedDevice_callback callback_proc)
Callback registration function.
unsigned long long BytesSent
@ CCSP_HAL_ETHSW_InterconnectPort2
enum _CCSP_HAL_ETHSW_ADMIN_STATUS CCSP_HAL_ETHSW_ADMIN_STATUS
@ CCSP_HAL_ETHSW_EthPort2
enum _CCSP_HAL_ETHSW_LINK_RATE CCSP_HAL_ETHSW_LINK_RATE
_CCSP_HAL_ETHSW_ADMIN_STATUS
int CcspHalEthSwInit(void)
int CcspHalEthSwSetAgingSpeed(CCSP_HAL_ETHSW_PORT PortId, int AgingSpeed)
enum _CCSP_HAL_ETHSW_DUPLEX_MODE CCSP_HAL_ETHSW_DUPLEX_MODE
@ CCSP_HAL_ETHSW_DUPLEX_Auto
enum _CCSP_HAL_ETHSW_PORT * PCCSP_HAL_ETHSW_PORT
int eth_port
which external port the device attached to. index start from 0
@ CCSP_HAL_ETHSW_AdminTest
int CcspHalEthSwGetEthPortStats(CCSP_HAL_ETHSW_PORT PortId, PCCSP_HAL_ETH_STATS pStats)
void(* fpEthWanLink_Down)()
int CcspHalEthSwLocatePortByMacAddress(unsigned char *mac, int *port)
@ CCSP_HAL_ETHSW_LINK_Down
@ CCSP_HAL_ETHSW_EthPort7
struct __appCallBack appCallBack
unsigned long BroadcastPacketsReceived
struct _CCSP_HAL_ETH_STATS CCSP_HAL_ETH_STATS
_CCSP_HAL_ETHSW_LINK_STATUS
enum _CCSP_HAL_ETHSW_DUPLEX_MODE * PCCSP_HAL_ETHSW_DUPLEX_MODE
unsigned long MulticastPacketsReceived
int CcspHalEthSwGetPortStatus(CCSP_HAL_ETHSW_PORT PortId, PCCSP_HAL_ETHSW_LINK_RATE pLinkRate, PCCSP_HAL_ETHSW_DUPLEX_MODE pDuplexMode, PCCSP_HAL_ETHSW_LINK_STATUS pStatus)
@ CCSP_HAL_ETHSW_LINK_100Mbps
unsigned long MulticastPacketsSent
@ CCSP_HAL_ETHSW_LINK_10Gbps
unsigned long PacketsSent
@ CCSP_HAL_ETHSW_EthPort4
@ CCSP_HAL_ETHSW_LINK_Disconnected
void GWP_RegisterEthWan_Callback(appCallBack *obj)
This function will get used to register RDKB functions to the callback pointers.
fpEthWanLink_Down pGWP_act_EthWanLinkDown
fpEthWanLink_Up pGWP_act_EthWanLinkUP
int GWP_GetEthWanInterfaceName(unsigned char *Interface, unsigned long maxSize)
This function will get used to retrieve the ETHWAN interface name. API returns 0 = success and 1 = fa...
unsigned long UnicastPacketsSent
enum _CCSP_HAL_ETHSW_LINK_RATE * PCCSP_HAL_ETHSW_LINK_RATE
enum _CCSP_HAL_ETHSW_LINK_STATUS * PCCSP_HAL_ETHSW_LINK_STATUS
@ CCSP_HAL_ETHSW_Processor1
@ CCSP_HAL_ETHSW_LINK_1Gbps
int CcspHalExtSw_getAssociatedDevice(unsigned long *output_array_size, eth_device_t **output_struct)
The HAL need to allocate array and return the array size by output_array_size.
unsigned long long BytesReceived
int CcspHalEthSwSetPortCfg(CCSP_HAL_ETHSW_PORT PortId, CCSP_HAL_ETHSW_LINK_RATE LinkRate, CCSP_HAL_ETHSW_DUPLEX_MODE DuplexMode)
int eth_vlanid
what vlan ID the the port tagged.
int CcspHalEthSwGetPortCfg(CCSP_HAL_ETHSW_PORT PortId, PCCSP_HAL_ETHSW_LINK_RATE pLinkRate, PCCSP_HAL_ETHSW_DUPLEX_MODE pDuplexMode)
unsigned long UnicastPacketsReceived
unsigned long DiscardPacketsReceived
@ CCSP_HAL_ETHSW_EthPort3
unsigned long DiscardPacketsSent
struct _eth_device eth_device_t
int CcspHalEthSwGetPortAdminStatus(CCSP_HAL_ETHSW_PORT PortId, PCCSP_HAL_ETHSW_ADMIN_STATUS pAdminStatus)
int GWP_GetEthWanLinkStatus()
@ CCSP_HAL_ETHSW_EthPort5
@ CCSP_HAL_ETHSW_EthPort8
@ CCSP_HAL_ETHSW_InterconnectPort1
@ CCSP_HAL_ETHSW_LINK_NULL
unsigned char eth_devMacAddress[6]
@ CCSP_HAL_ETHSW_EthPort6
int CcspHalExtSw_getEthWanPort(unsigned int *pPort)
int(* CcspHalExtSw_ethAssociatedDevice_callback)(eth_device_t *eth_dev)
This call back will be invoked when new Ethernet client come to associate to AP, or existing Ethernet...
enum _CCSP_HAL_ETHSW_LINK_STATUS CCSP_HAL_ETHSW_LINK_STATUS
@ CCSP_HAL_ETHSW_LINK_2_5Gbps
@ CCSP_HAL_ETHSW_DUPLEX_Half
unsigned long UnknownProtoPacketsReceived
struct _CCSP_HAL_ETH_STATS * PCCSP_HAL_ETH_STATS
_CCSP_HAL_ETHSW_DUPLEX_MODE
unsigned long PacketsReceived
enum _CCSP_HAL_ETHSW_PORT CCSP_HAL_ETHSW_PORT
@ CCSP_HAL_ETHSW_LINK_Auto
enum _CCSP_HAL_ETHSW_ADMIN_STATUS * PCCSP_HAL_ETHSW_ADMIN_STATUS
@ CCSP_HAL_ETHSW_LINK_10Mbps
int CcspHalExtSw_setEthWanEnable(unsigned char Flag)
@ CCSP_HAL_ETHSW_LINK_5Gbps
int CcspHalExtSw_getEthWanEnable(unsigned char *pFlag)
@ CCSP_HAL_ETHSW_AdminDown
void(* fpEthWanLink_Up)()
@ CCSP_HAL_ETHSW_DUPLEX_Full
@ CCSP_HAL_ETHSW_Processor2
_CCSP_HAL_ETHSW_LINK_RATE
@ CCSP_HAL_ETHSW_MgmtPort