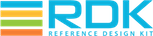 |
RDK-B
|
Go to the documentation of this file.
59 #ifndef __VLAN_ETH_HAL_H__
60 #define __VLAN_ETH_HAL_H__
67 #define ULONG unsigned long
71 #define BOOL unsigned char
79 #define UCHAR unsigned char
87 #define UINT unsigned int
107 #define RETURN_ERR -1
111 #define VLAN_ETH_HAL_MAJOR_VERSION 1 // This is the major verion of this HAL.
112 #define VLAN_ETH_HAL_MINOR_VERSION 0 // This is the minor verson of the HAL.
113 #define VLAN_ETH_HAL_MAINTENANCE_VERSION 1 // This is the maintenance version of the HAL.
int vlan_eth_hal_getInterfaceStatus(const char *vlan_ifname, vlan_interface_status_e *status)
int vlan_eth_hal_deleteInterface(const char *vlan_ifname)
unsigned int skbEthPriorityMark
vlan_interface_status_e Status
vlan_skb_config_t * skb_config
struct _VLAN_SKB_CONFIG vlan_skb_config_t
int vlan_eth_hal_configureInterface(vlan_configuration_t *config)
struct _vlan_configuration vlan_configuration_t
unsigned int skbMarkingNumOfEntries
unsigned char doReconfigure