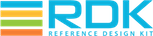 |
RDK-B
|
Go to the documentation of this file.
39 #ifndef _BRIDGE_UTIL_OEM_H
40 #define _BRIDGE_UTIL_OEM_H
50 #include "OvsAgentApi.h"
57 #define BRIDGE_UTIL_LOG_FNAME "/rdklogs/logs/bridgeUtils.log"
59 #define GRE_HANDLER_SCRIPT "/etc/utopia/service.d/service_multinet/handle_gre.sh"
61 #define TOTAL_IFLIST_SIZE 1024
62 #define BRIDGE_NAME_SIZE 64
63 #define IFACE_NAME_SIZE 64
65 #define IFLIST_SIZE 256
66 #define MAX_LOG_BUFF_SIZE 1024
69 #define INTERFACE_EXIST 0
70 #define INTERFACE_NOT_EXIST -1
91 #define bridge_util_log(fmt ...) {\
92 snprintf(log_buff, MAX_LOG_BUFF_SIZE-1,fmt);\
94 snprintf(log_buff, MAX_LOG_BUFF_SIZE-1,fmt);\
95 utc_time = time(NULL);\
96 timeinfo = gmtime(&utc_time);\
97 snprintf(log_msg_wtime, MAX_LOG_BUFF_SIZE+TIMESTAMP-1,"%04d-%02d-%02d %02d:%02d:%02d ::: %s",timeinfo->tm_year+1900,timeinfo->tm_mon+1,timeinfo->tm_mday,timeinfo->tm_hour,timeinfo->tm_min,timeinfo->tm_sec,log_buff);\
98 fprintf(logFp,"%s", log_msg_wtime);\
int checkIfExists(char *iface_name)
Check if interface is created.
int need_switch_gw_refresh
BridgeOpr
BridgeUtils Operations for BridgeUtils API's.
char * getVendorIfaces()
Provides vendor interface information for creating/updating/deleting bridge.
int HandlePostConfigVendor(bridgeDetails *bridgeInfo, int Config)
Provides OEM/SOC specific changes which needs to be configured after creating/updating/deleting bridg...
struct bridgeDetails bridgeDetails
Config
List of Configurations for BridgeUtils API's.
char VirtualParentIfname[64]
int checkIfExistsInBridge(char *iface_name, char *bridge_name)
Check if interface is attached to bridge.
int updateBridgeInfo(bridgeDetails *bridgeInfo, char *ifNameToBeUpdated, int Opr, int type)
Provides generic changes which needs to be configured after creating/updating/deleting bridge.
char log_msg_wtime[1024+64]
int HandlePreConfigVendor(bridgeDetails *bridgeInfo, int Config)
Provides OEM/SOC specific changes which needs to be configured before creating/updating/deleting brid...
void removeIfaceFromList(char *str, const char *sub)
Remove interface from the list of interfaces.
char primaryBridgeName[64]
INTERFACE_TYPE
List of Interface types for BridgeUtils API's.
#define MAX_LOG_BUFF_SIZE