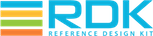 |
RDK-B
|
Go to the documentation of this file.
67 #ifndef __MOCA_HAL_H__
68 #define __MOCA_HAL_H__
72 #define ULONG unsigned long
76 #define BOOL unsigned char
84 #define UCHAR unsigned char
92 #define UINT unsigned int
107 #ifndef STATUS_SUCCESS
108 #define STATUS_SUCCESS 0
111 #ifndef STATUS_FAILURE
112 #define STATUS_FAILURE -1
115 #ifndef STATUS_NOT_AVAILABLE
116 #define STATUS_NOT_AVAILABLE -2
137 #define kMoca_MaxCpeList 256
138 #define kMoca_MaxMocaNodes 16
139 #define MAC_PADDING 12
141 #define STATUS_INPROGRESS -1
142 #define STATUS_NO_NODE -2
143 #define STATUS_INVALID_PROBE -3
144 #define STATUS_INVALID_CHAN -4
172 STAT_FAIL_BADCHANNEL,
173 STAT_FAIL_NOEVMPROBE,
182 ULONG InstanceNumber;
198 BOOL AutoPowerControlEnable;
245 CHAR NetworkCoordinatorMACAddress[18];
358 char DestinationMACAddress[18];
unsigned char QAM256Capable
unsigned char ACATrapCompleted
int moca_getIfAcaConfig(int interfaceIndex, moca_aca_cfg_t *acaCfg)
unsigned long DiscardPacketsSent
int moca_FreqMaskToValue(unsigned char *mask)
int moca_IfGetStats(unsigned long ifIndex, moca_stats_t *pmoca_stats)
unsigned long ExtAggrAverageTx
int moca_GetFullMeshRates(unsigned long ifIndex, moca_mesh_table_t *pDeviceArray, unsigned long *pulCount)
int moca_IfGetStaticInfo(unsigned long ifIndex, moca_static_info_t *pmoca_static_info)
@ IF_STATUS_LowerLayerDown
unsigned long PacketsSent
int moca_GetIfConfig(unsigned long ifIndex, moca_cfg_t *pmoca_config)
unsigned long MulticastPacketsReceived
unsigned long BroadcastPacketsReceived
unsigned char MaxIngressBWThresholdReached
unsigned long BytesReceived
int moca_GetNumAssociatedDevices(unsigned long ifIndex, unsigned long *pulCount)
unsigned long TxBcastRate
unsigned long MaxEgressBW
unsigned long RxErroredAndMissedPackets
unsigned long EgressNodeID
unsigned long TxPowerControlReduction
int moca_GetMocaCPEs(unsigned long ifIndex, moca_cpe_t *cpes, int *pnum_cpes)
unsigned long PacketsReceived
struct moca_assoc_pnc_info moca_assoc_pnc_info_t
unsigned long ExtAggrAverageRx
int moca_SetIfConfig(unsigned long ifIndex, moca_cfg_t *pmoca_config)
unsigned long CurrentOperFreq
int moca_IfGetExtCounter(unsigned long ifIndex, moca_mac_counters_t *pmoca_mac_counters)
int moca_GetFlowStatistics(unsigned long ifIndex, moca_flow_table_t *pDeviceArray, unsigned long *pulCount)
unsigned long ErrorsReceived
int moca_GetAssociatedDevices(unsigned long ifIndex, moca_associated_device_t **ppdevice_array)
unsigned long IngressNodeID
unsigned long TxRateVlper
unsigned char PacketAggregationCapability
unsigned long UnicastPacketsSent
unsigned char PacketAggregationCapability
int moca_cancelIfAca(int interfaceIndex)
unsigned char moca_HardwareEquipped(void)
void moca_associatedDevice_callback_register(moca_associatedDevice_callback callback_proc)
int moca_getIfAcaStatus(int interfaceIndex, moca_aca_stat_t *pacaStat)
unsigned long mocaNodeIndex
int(* moca_associatedDevice_callback)(unsigned long ifIndex, moca_associated_device_t *moca_dev)
unsigned long NumberOfClients
unsigned long RxBcastRate
int moca_GetResetCount(unsigned long *resetcnt)
unsigned char QAM256Capable
unsigned long LastOperFreq
unsigned char PreferredNC
unsigned long NetworkCoordinator
unsigned long PeakDataRate
int moca_setIfAcaConfig(int interfaceIndex, moca_aca_cfg_t acaCfg)
int moca_IfGetDynamicInfo(unsigned long ifIndex, moca_dynamic_info_t *pmoca_dynamic_info)
unsigned long TxBcastRate
unsigned long MaxIngressBW
unsigned long DiscardPacketsReceived
int moca_IfGetExtAggrCounter(unsigned long ifIndex, moca_aggregate_counters_t *pmoca_aggregate_counts)
unsigned long BroadcastPacketsSent
unsigned long UnicastPacketsReceived
unsigned long UnknownProtoPacketsReceived
unsigned char mocaNodePreferredNC
unsigned char PrivacyEnabled
unsigned long NumberOfConnectedClients
unsigned long mocaNodeMocaversion
unsigned long MulticastPacketsSent
unsigned long TxBcastPowerReduction
int moca_getIfScmod(int interfaceIndex, int *pnumOfEntries, moca_scmod_stat_t **ppscmodStat)
unsigned char MaxEgressBWThresholdReached
unsigned long FlowTimeLeft