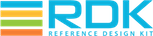 |
RDK-B
|
Go to the documentation of this file.
32 #ifndef __WIFI_HAL_GENERIC_H__
33 #define __WIFI_HAL_GENERIC_H__
46 #define ULLONG unsigned long long
50 #define ULONG unsigned long
54 #define USHORT unsigned short
58 #define BOOL unsigned char
66 #define UCHAR unsigned char
74 #define UINT unsigned int
98 #define WIFI_HAL_SUCCESS 0
99 #define WIFI_HAL_ERROR -1
100 #define WIFI_HAL_INTERNAL_ERROR -2
101 #define WIFI_HAL_UNSUPPORTED -3
102 #define WIFI_HAL_INVALID_ARGUMENTS -4
103 #define WIFI_HAL_INVALID_VALUE -5
106 #ifndef RADIO_INDEX_1
107 #define RADIO_INDEX_1 1
108 #define RADIO_INDEX_2 2
109 #define RADIO_INDEX_3 3
120 #define AP_INDEX_10 10
121 #define AP_INDEX_11 11
122 #define AP_INDEX_12 12
123 #define AP_INDEX_13 13
124 #define AP_INDEX_14 14
125 #define AP_INDEX_15 15
126 #define AP_INDEX_16 16
127 #define AP_INDEX_17 17
128 #define AP_INDEX_18 18
129 #define AP_INDEX_19 19
130 #define AP_INDEX_20 20
131 #define AP_INDEX_21 21
132 #define AP_INDEX_22 22
133 #define AP_INDEX_23 23
134 #define AP_INDEX_24 24
138 #ifdef WIFI_HAL_VERSION_3
139 #define MAX_NUM_RADIOS 3
141 #define MAX_NUM_RADIOS 2
144 #define MAX_NUM_VAP_PER_RADIO 8
146 #define MAC_STR_LEN 18
147 #ifndef ETHER_ADDR_LEN
148 #define ETHER_ADDR_LEN 6
151 #define ACL_MACADDR_SIZE 18
152 #define ACL_MACFLT_NUM 16
153 #define MAC_LIST_SIZE ( (ACL_MACADDR_SIZE * WL_MACADDR_SIZE) + 2 )
154 #define ACL_MAC_ARRAY_MAX 512
155 #define ASSOC_MAC_ARRAY_MAX 1024
156 #define SUPPORTED_STANDARDS_RADIO2_4GHZ "b,g,n"
157 #define SUPPORTED_STANDARDS_RADIO5GHZ "a,n,ac"
158 #define RESTORE_CNFG_FILE_NAME "/data/.nvram_restore_cfg.txt"
159 #define NVRAM_LINE_MAX (1024)
162 #define WIFI_HAL_MAJOR_VERSION 3
163 #define WIFI_HAL_MINOR_VERSION 0
164 #define WIFI_HAL_MAINTENANCE_VERSION 1
165 #define WIFI_HAL_VERSION (WIFI_HAL_MAJOR_VERSION *1000+ WIFI_HAL_MINOR_VERSION *10+ WIFI_HAL_MAINTENANCE_VERSION)
167 #define MAX_NUM_TWT_SESSION 50
168 #define MAX_STA_PER_SESSION 100
170 #define MAX_RU_ALLOCATIONS 74
177 #define CONN_RECONN_AFTER_INACTIVITY 3
179 #define KI1_VER_MASK 0xf8
181 #define KI1_PW_KEY 0x08
182 #define KI1_INSTALL 0x40
185 #define KI1_MSG1_BITS (KI1_PW_KEY | KI1_ACK)
186 #define KI1_MSG3_BITS (KI1_PW_KEY | KI1_INSTALL|KI1_ACK)
189 #define KI0_SECURE 0x02
190 #define KI0_ENCR 0x10
192 #define KI0_MSG3_BITS (KI0_MIC | KI0_SECURE | KI0_ENCR)
193 #define KI0_MSG4_BITS (KI0_MIC | KI0_SECURE)
195 #define KEY_MSG_1_OF_4(msg) \
196 ((((msg)->key_info[1] & KI1_VER_MASK) == KI1_MSG1_BITS) && ((msg)->key_info[0] == 0))
198 #define KEY_MSG_2_OF_4(msg) \
199 ((((msg)->key_info[1] & KI1_VER_MASK) == KI1_PW_KEY) && ((msg)->key_info[0] == KI0_MIC))
201 #define KEY_MSG_3_OF_4(msg) \
202 ((((msg)->key_info[1] & KI1_VER_MASK) == KI1_MSG3_BITS) && ((msg)->key_info[0] == KI0_MSG3_BITS))
204 #define KEY_MSG_4_OF_4(msg) \
205 ((((msg)->key_info[1] & KI1_VER_MASK) == KI1_PW_KEY) && ((msg)->key_info[0] == KI0_MSG4_BITS))
233 unsigned char ie[256];
275 #define MAX_NUM_FREQ_BAND 4
293 #define MAX_CHANNELS 64
317 #define MAXNUMBEROFTRANSMIPOWERSUPPORTED 21
325 }
__attribute__((packed)) wifi_radio_trasmitPowerSupported_list_t;
346 #ifdef WIFI_HAL_RSN_SELECTOR
347 #undef WIFI_HAL_RSN_SELECTOR
349 #define WIFI_HAL_RSN_SELECTOR(a, b, c, d) \
350 ((((unsigned int) (a)) << 24) | (((unsigned int) (b)) << 16) | (((unsigned int) (c)) << 8) | \
353 #define WIFI_HAL_RSN_CIPHER_SUITE_NONE WIFI_HAL_RSN_SELECTOR(0x00, 0x0f, 0xac, 0)
354 #define WIFI_HAL_RSN_CIPHER_SUITE_TKIP WIFI_HAL_RSN_SELECTOR(0x00, 0x0f, 0xac, 2)
356 #define WIFI_HAL_RSN_CIPHER_SUITE_WRAP WIFI_HAL_RSN_SELECTOR(0x00, 0x0f, 0xac, 3)
358 #define WIFI_HAL_RSN_CIPHER_SUITE_CCMP WIFI_HAL_RSN_SELECTOR(0x00, 0x0f, 0xac, 4)
359 #define WIFI_HAL_RSN_CIPHER_SUITE_AES_128_CMAC WIFI_HAL_RSN_SELECTOR(0x00, 0x0f, 0xac, 6)
360 #define WIFI_HAL_RSN_CIPHER_SUITE_NO_GROUP_ADDRESSED WIFI_HAL_RSN_SELECTOR(0x00, 0x0f, 0xac, 7)
361 #define WIFI_HAL_RSN_CIPHER_SUITE_GCMP WIFI_HAL_RSN_SELECTOR(0x00, 0x0f, 0xac, 8)
362 #define WIFI_HAL_RSN_CIPHER_SUITE_GCMP_256 WIFI_HAL_RSN_SELECTOR(0x00, 0x0f, 0xac, 9)
363 #define WIFI_HAL_RSN_CIPHER_SUITE_CCMP_256 WIFI_HAL_RSN_SELECTOR(0x00, 0x0f, 0xac, 10)
364 #define WIFI_HAL_RSN_CIPHER_SUITE_BIP_GMAC_128 WIFI_HAL_RSN_SELECTOR(0x00, 0x0f, 0xac, 11)
365 #define WIFI_HAL_RSN_CIPHER_SUITE_BIP_GMAC_256 WIFI_HAL_RSN_SELECTOR(0x00, 0x0f, 0xac, 12)
366 #define WIFI_HAL_RSN_CIPHER_SUITE_BIP_CMAC_256 WIFI_HAL_RSN_SELECTOR(0x00, 0x0f, 0xac, 13)
368 #define WIFI_CIPHER_CAPA_ENC_WEP40 0x00000001
369 #define WIFI_CIPHER_CAPA_ENC_WEP104 0x00000002
370 #define WIFI_CIPHER_CAPA_ENC_TKIP 0x00000004
371 #define WIFI_CIPHER_CAPA_ENC_CCMP 0x00000008
372 #define WIFI_CIPHER_CAPA_ENC_WEP128 0x00000010
373 #define WIFI_CIPHER_CAPA_ENC_GCMP 0x00000020
374 #define WIFI_CIPHER_CAPA_ENC_GCMP_256 0x00000040
375 #define WIFI_CIPHER_CAPA_ENC_CCMP_256 0x00000080
376 #define WIFI_CIPHER_CAPA_ENC_BIP 0x00000100
377 #define WIFI_CIPHER_CAPA_ENC_BIP_GMAC_128 0x00000200
378 #define WIFI_CIPHER_CAPA_ENC_BIP_GMAC_256 0x00000400
379 #define WIFI_CIPHER_CAPA_ENC_BIP_CMAC_256 0x00000800
380 #define WIFI_CIPHER_CAPA_ENC_GTK_NOT_USED 0x00001000
649 #define MAXIFACENAMESIZE 64
668 wifi_radio_csi_capabilities_t
csi;
690 unsigned int phy_index;
844 #define MAX_SUB_CARRIERS 256
845 #define MAX_PILOTS 26
@ WIFI_EVENT_DFS_RADAR_DETECTED
wifi_ieee80211Variant_t
Wifi 802.11 variant Types.
unsigned char BandSteeringSupported
unsigned int numDevicesInSession
@ wifi_operating_env_non_country
int wifi_createHostApdConfig(int apIndex, unsigned char createWpsCfg)
Creates configuration variables needed for WPA/WPS.
@ WIFI_CHANNELBANDWIDTH_40MHZ
unsigned int wakeTime_uSec
wifi_bitrate_t
Wifi supported bitrates.
@ wifi_connection_status_disabled
char wifi_interface_name_t[32]
char cli_InterferenceSources[64]
unsigned int numTwtSession
unsigned long cli_MultipleRetryCount
This structure hold the information about the wifi interface.
@ wifi_connection_status_ap_not_found
unsigned int wifi_carrier_data_t[8][4]
unsigned char cli_AllocatedDownlinkRuNum
unsigned long long cli_Associations
unsigned int cli_Disassociations
@ WIFI_CHANNELBANDWIDTH_80MHZ
unsigned char sessionPaused
unsigned char mcast2ucastSupported
wifi_frame_info_t frame_info
unsigned int numcountrySupported
@ WIFI_CHANNELBANDWIDTH_80_80MHZ
wifi_interface_name_t interface_name
unsigned int cipherSupported
unsigned short valid_mask
@ WIFI_CHANNELBANDWIDTH_20MHZ
@ wifi_access_category_best_effort
unsigned char cli_ChannelStateInformation
wifi_access_category_t access_category
int wifi_getHalCapability(wifi_hal_capability_t *cap)
Get HAL Capabilities.
This structure hold the information about the wifi interface.
unsigned int rdk_radio_index
@ wifi_access_category_background
unsigned char zeroDFSSupported
unsigned int cli_Retransmissions
wifi_twt_individual_params_t individual
int wifi_createInitialConfigFiles()
This function creates wifi configuration files.
wifi_freq_bands_t
Wifi Frequency Band Types.
@ wifi_connection_status_disconnected
@ wifi_twt_agreement_type_individual
wifi_channelBandwidth_t
Wifi Channel Bandwidth Types.
unsigned long cli_RetryCount
unsigned int cli_activeNumSpatialStreams
wifi_twt_dev_info_t cli_TwtParams
unsigned long cli_DataFramesSentAck
mac_address_t cli_MACAddress
unsigned char subchannels
unsigned long long time_stamp
@ wifi_operating_env_indoor
wifi_carrier_data_t wifi_csi_matrix_t[256]
unsigned int cli_LastDataUplinkRate
unsigned int cli_AuthenticationFailures
wifi_twt_agreement_type_t
Wifi TWT agreement type.
#define MAX_NUM_VAP_PER_RADIO
@ wifi_operating_env_outdoor
unsigned int wakeInterval_uSec
#define MAX_NUM_TWT_SESSION
#define MAX_STA_PER_SESSION
unsigned int numSupportedFreqBand
@ wifi_connection_status_connected
#define MAX_NUM_FREQ_BAND
This structure hold the information about the wifi interface.
wifi_interface_name_t bridge_name
unsigned int cli_MaxDownlinkRate
wifi_platform_property_t wifi_prop
unsigned int wakeInterval_uSec
unsigned int wifi_radio_index_t
wifi_evm_data_t wifi_evm_matrix_t[26]
wifi_dl_mu_stats_t cli_UplinkMuStats
unsigned char DCSSupported
unsigned int cli_LastDataDownlinkRate
unsigned long cli_RetransCount
int wifi_init()
This function call initializes all Wi-Fi radios.
unsigned int numberOfElements
wifi_dl_mu_type_t cli_DownlinkMuType
@ wifi_access_category_video
wifi_streams_rssi_t nr_rssi
@ wifi_access_category_voice
wifi_ul_mu_type_t cli_UpinkMuType
#define MAXNUMBEROFTRANSMIPOWERSUPPORTED
@ WIFI_DL_MU_TYPE_OFDMA_MIMO
unsigned long cli_ErrorsSent
struct _wifi_frame_info wifi_frame_info_t
This structure hold the information about the wifi interface.
unsigned long cli_FailedRetransCount
@ WIFI_FREQUENCY_2_4_BAND
unsigned char autoChannelSupported
struct _wifi_associated_dev3 wifi_associated_dev3_t
This structure hold the information about the wifi interface.
wifi_access_category_t
Wifi access category (AC) type.
#define MAX_RU_ALLOCATIONS
int wifi_streams_rssi_t[8]
unsigned long cli_PacketsReceived
char cli_OperatingChannelBandwidth[64]
unsigned char cli_AuthenticationState
wifi_twt_operation_t operation
unsigned int wakeDuration_uSec
unsigned int minWakeDuration_uSec
wifi_csi_data_t * cli_CsiData
@ WIFI_CHANNELBANDWIDTH_160MHZ
int wifi_factoryReset()
Clears internal variables to implement a factory reset of the Wi-Fi subsystem.
unsigned long cli_BytesReceived
wifi_ul_mu_stats_t cli_DownlinkMuStats
unsigned long cli_DataFramesSentNoAck
unsigned char soudingFrameSupported
@ WIFI_EVENT_CHANNELS_CHANGED
unsigned char r0r1_key_t[16]
wifi_twt_broadcast_params_t broadcast
int wifi_down()
Turns off transmit power for the entire Wifi subsystem, for all radios.
wifi_hal_version_t version
unsigned char trigger_enabled
int wifi_stopHostApd()
Stops hostapd.
int wifi_startHostApd()
Starts hostapd.
wifi_evm_matrix_t evm_matrix
int wifi_setLED(int radioIndex, unsigned char enable)
Set the system LED status.
unsigned long cli_BytesSent
unsigned int maxNumberVAPs
unsigned long cli_PacketsSent
wifi_twt_params_t twtParameters
unsigned char cli_AllocatedUplinkRuNum
wifi_radio_csi_capabilities_t csi
unsigned char wifi_evm_data_t[4][8]
struct _wifi_csi_data wifi_csi_data_t
This structure hold the information about the wifi interface.
wifi_csi_matrix_t csi_matrix
wifi_twt_params_t twt_params
unsigned int cli_MaxUplinkRate
unsigned char mac_address_t[6]
@ wifi_twt_agreement_type_broadcast
char cli_OperatingStandard[64]
int wifi_reset()
Resets the Wifi subsystem. This includes reset of all Access Point variables.
wifi_twt_agreement_type_t agreement