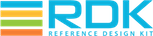 |
RDK-B
|
Go to the documentation of this file.
20 #ifndef __WIFI_HAL_CLIENTMGT_H__
21 #define __WIFI_HAL_CLIENTMGT_H__
723 #define MAX_KEY_HOLDERS 8
INT wifi_getApAssociatedDevicesHighWatermark(INT apIndex, UINT *output)
Maximum number of associated devices that have ever associated with the access point concurrently sin...
INT wifi_getBSSTransitionActivation(UINT apIndex, BOOL *activate)
unsigned int uiEAPRequestRetries
INT wifi_pushApFastTransitionConfig(INT apIndex, wifi_FastTransitionConfig_t *ftData)
INT wifi_setFTOverDSActivated(INT apIndex, BOOL *activate)
Set the Fast Transition over DS activated value. See 802.11-2016 section 13.3.
INT wifi_setNeighborReportActivation(UINT apIndex, BOOL activate)
INT wifi_getApAssociatedDevicesHighWatermarkThresholdReached(INT apIndex, UINT *output)
Get the number of times the current total number of associated device has reached the HighWatermarkTh...
wifi_fastTrasitionSupport_t
INT wifi_getBandSteeringLog(INT record_index, ULONG *pSteeringTime, CHAR *pClientMAC, INT *pSourceSSIDIndex, INT *pDestSSIDIndex, INT *pSteeringReason)
To get the band steering log.
INT wifi_getFTMobilityDomainID(INT apIndex, UCHAR mobilityDomain[2])
Get the Fast Transition Mobility Domain value. See 802.11-2016 section 13.3.
INT wifi_getBandSteeringApGroup(char *output_ApGroup)
To get Band Steering Access Point group.
INT wifi_setApAssociatedDevicesHighWatermarkThreshold(INT apIndex, UINT Threshold)
Set the HighWatermarkThreshold value, that is lesser than or equal to MaxAssociatedDevices.
INT wifi_setBandSteeringOverloadInactiveTime(INT radioIndex, INT overloadInactiveTime)
To set the inactivity time (in seconds) for steering under overload condition.
unsigned int uiEAPOLKeyRetries
INT wifi_setBandSteeringBandUtilizationThreshold(INT radioIndex, INT buThreshold)
To set the band steering BandUtilizationThreshold parameters.
unsigned int uiEAPIdentityRequestTimeout
INT wifi_getApAssociatedDevicesHighWatermarkThreshold(INT apIndex, UINT *output)
Get the HighWatermarkThreshold value, that is lesser than or equal to MaxAssociatedDevices.
INT wifi_setBandSteeringPhyRateThreshold(INT radioIndex, INT prThreshold)
To set the band steering physical modulation rate threshold parameters.
INT wifi_setFTMobilityDomainID(INT apIndex, UCHAR mobilityDomain[2])
Set the Fast Transition Mobility Domain value. See 802.11-2016 section 13.3.
INT wifi_getNeighborReportActivation(UINT apIndex, BOOL *activate)
INT wifi_setFTR1KeyHolderID(INT apIndex, UCHAR *keyHolderID)
Set the Fast Transition R1 Key Holder ID value. See 802.11-2016 section 13.3.
INT wifi_setEAP_Param(UINT apIndex, UINT value, char *param)
Get the Fast Transition over DS activated value. @description Set the EAP authentication and EAPOL ...
INT wifi_getFTR0KeyLifetime(INT apIndex, UINT *lifetime)
Get the Fast Transition R0 Key Lifetime value. See 802.11-2016 section 13.4.2.
INT wifi_getBandSteeringRSSIThreshold(INT radioIndex, INT *pRssiThreshold)
To read the band steering RSSIThreshold parameters.
INT wifi_setBandSteeringApGroup(char *ApGroup)
To set Band Steering Access Point group.
unsigned int uiEAPOLKeyTimeout
INT wifi_setFTR0KeyLifetime(INT apIndex, UINT *lifetime)
Set the Fast Transition R0 Key Lifetime value. See 802.11-2016 section 13.4.2.
Set the Fast Transition capability to disabled, full FT.
wifi_fastTrasitionSupport_t support
INT wifi_getApAssociatedDevicesHighWatermarkDate(INT apIndex, ULONG *output_in_seconds)
Get Date and Time at which the maximum number of associated devices ever associated with the access p...
INT wifi_setBandSteeringIdleInactiveTime(INT radioIndex, INT idleInactiveTime)
To set the inactivity time (in seconds) for steering under Idle condition.
INT wifi_getEAP_Param(UINT apIndex, wifi_eap_config_t *output)
INT wifi_setFTR0KeyHolderID(INT apIndex, UCHAR *keyHolderID)
Set the Fast Transition R0 Key Holder ID value. See 802.11-2016 section 13.3.
INT wifi_setBandSteeringRSSIThreshold(INT radioIndex, INT rssiThreshold)
To set the band steering RSSIThreshold parameters.
INT wifi_getFTResourceRequestSupported(INT apIndex, BOOL *supported)
Get the Fast Transition Resource Request Support value. See 802.11-2016 section 13....
INT wifi_getFTR1KeyHolderID(INT apIndex, UCHAR *keyHolderID)
Get the Fast Transition R1 Key Holder ID value. See 802.11-2016 section 13.3.
struct _wifi_eap_config_t wifi_eap_config_t
Set the Fast Transition capability to disabled, full FT.
INT wifi_setBandSteeringEnable(BOOL enable)
To turn on/off Band steering.
unsigned char r0r1_key_t[16]
INT wifi_getBandSteeringPhyRateThreshold(INT radioIndex, INT *pPrThreshold)
To read the band steering physical modulation rate threshold parameters.
mac_address_t r1KeyHolder
INT wifi_getFTOverDSActivated(INT apIndex, BOOL *activate)
unsigned int uiEAPRequestTimeout
INT wifi_setFastBSSTransitionActivated(INT apIndex, UCHAR activate)
INT wifi_getBandSteeringEnable(BOOL *enable)
To get Band Steering enable status.
unsigned int uiEAPIdentityRequestRetries
INT wifi_getBandSteeringIdleInactiveTime(INT radioIndex, INT *idleInactiveTime)
To read the inactivity time (in seconds) for steering under Idle condition.
INT wifi_getBSSTransitionActivated(INT apIndex, BOOL *activate)
Get the Fast Transition capability value.
unsigned char mac_address_t[6]
INT wifi_setFTResourceRequestSupported(INT apIndex, BOOL *supported)
Set the Fast Transition Resource Request Support value. See 802.11-2016 section 13....
INT wifi_getBandSteeringBandUtilizationThreshold(INT radioIndex, INT *pBuThreshold)
To set and read the band steering BandUtilizationThreshold parameters.
INT wifi_getBandSteeringOverloadInactiveTime(INT radioIndex, INT *overloadInactiveTime)
To read the inactivity time (in seconds) for steering under overload condition.
INT wifi_setBSSTransitionActivation(UINT apIndex, BOOL activate)
Set the BTM capability to activated or deactivated, same as enabled or disabled. The word "activated"...
INT wifi_getFTR0KeyHolderID(INT apIndex, UCHAR *keyHolderID)
Get the Fast Transition R0 Key Holder ID value. See 802.11-2016 section 13.3.