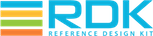 |
RDK-B
|
Go to the documentation of this file.
19 #ifndef __WIFI_HAL_AP_H__
20 #define __WIFI_HAL_AP_H__
87 wifi_authMethod_t auth_method[16];
96 wifi_eapMethod_t eap_method[16];
101 wifi_naiRealm_t nai_realm_tuples[20];
113 wifi_venueName_t venueNameTuples[16];
124 wifi_plmn_t plmn[16];
125 }
__attribute__((packed))wifi_3gpp_plmn_list_information_element_t;
139 wifi_domainNameTuple_t domainNameTuple[4];
148 wifi_ouiDuple_t ouiDuple[32];
157 UCHAR wifiRoamingConsortiumOui[3][15+1];
180 wifi_HS2_OperatorNameDuple_t operatorNameDuple[16];
230 wifi_HS2_Proto_Port_Tuple_t protoPortTuple[16];
277 unsigned char key_info[2];
279 unsigned char replay[8];
280 unsigned char nonce[32];
281 unsigned char init_vector[16];
282 unsigned char rsc[8];
283 unsigned char key_id[8];
284 unsigned char mic[16];
286 unsigned char data[0];
300 unsigned char data[0];
314 unsigned char data[0];
398 #ifdef WIFI_HAL_VERSION_3_PHASE2
557 #ifdef WIFI_HAL_VERSION_3_PHASE2
619 #ifdef WIFI_HAL_VERSION_3_PHASE2
641 #ifdef WIFI_HAL_VERSION_3_PHASE2
1611 #ifdef WIFI_HAL_VERSION_3_PHASE2
1917 #ifdef WIFI_HAL_VERSION_3_PHASE2
1986 #define WIFI_ETH_TYPE_IP 0x0800
1987 #define WIFI_ETH_TYPE_ARP 0x0806
1988 #define WIFI_ETH_TYPE_REVARP 0x8035
1989 #define WIFI_ETH_TYPE_VLAN 0x8100
1990 #define WIFI_ETH_TYPE_LOOPBACK 0x9000
1991 #define WIFI_ETH_TYPE_IP6 0x86DD
1992 #define WIFI_ETH_TYPE_EAPOL 0x888e
2063 #define GAS_CFG_TYPE_SUPPORTED 1
2372 #define WIFI_AP_MAX_WPSPIN_LEN 9
2395 #ifdef WIFI_HAL_VERSION_3_PHASE2
2398 unsigned char ip[45];
2403 #ifdef WIFI_HAL_VERSION_3_PHASE2
2406 unsigned char s_ip[45];
2464 #if defined(WIFI_HAL_VERSION_3)
2518 int capabilityInfoLength;
2524 int realmInfoLength;
2558 #define WIFI_AP_MAX_SSID_LEN 33
2571 wifi_vap_security_t security;
2585 char beaconRateCtl[32];
2590 #define WIFI_BRIDGE_NAME_LEN 32
INT wifi_getApMaxAssociatedDevices(INT apIndex, UINT *output)
Get maximum associated devices with the Access Point index.
INT wifi_getApWmmUapsdEnable(INT apIndex, BOOL *output)
Indicates whether U-APSD support is currently enabled.
INT wifi_setApWmmOgAckPolicy(INT apIndex, INT cla, BOOL ackPolicy)
Sets the WMM ACK policy on the hardware.
@ wifi_eapol_type_eapol_logoff
INT wifi_pushApRoamingConsortiumElement(INT apIndex, wifi_roamingConsortiumElement_t *infoElement)
INT wifi_getApWpsConfiguration(INT ap_index, wifi_wps_t *wpsConfig)
void wifi_apDisassociatedDevice_callback_register(wifi_apDisassociatedDevice_callback callback_proc)
Callback registration function.
BOOL PauseForServerResponse
wifi_bitrate_t
Wifi supported bitrates.
INT(* wifi_vapstatus_callback)(INT apIndex, wifi_vapstatus_t status)
This call back will be invoked when VAP status changes.
INT wifi_getApIsolationEnable(INT apIndex, BOOL *output)
Get Access Point isolation value.
ULONG wifi_MulticastPacketsReceived
INT wifi_getApAclDevices(INT apIndex, CHAR *macArray, UINT buf_size)
Get the ACL MAC list per Access Point.
BOOL rapidReconnectEnable
wifi_ipAddressAvailabality_t ipAddressInfo
INT wifi_getApDASRadiusServer(INT apIndex, CHAR *IP_output, UINT *Port_output, CHAR *RadiusdasSecret_output)
INT(* wifi_csi_callback)(mac_address_t mac_addr, wifi_csi_data_t *csi_data)
INT wifi_pushApInterworkingElement(INT apIndex, wifi_InterworkingElement_t *infoEelement)
wifi_onboarding_methods_t methods
INT wifi_getApSsidAdvertisementEnable(INT apIndex, BOOL *output_bool)
Indicates whether or not beacons include the SSID name.
UINT ResponseBufferingTime
@ WIFI_MGMT_FRAME_TYPE_DISASSOC
INT wifi_setBssLoad(INT apIndex, BOOL enabled)
wifi_passpoint_settings_t passpoint
ULONG cli_DataFramesSentNoAck
INT wifi_setApWmmUapsdEnable(INT apIndex, BOOL enable)
Enables/disables Automatic Power Save Delivery on the hardwarwe for this Access Point.
INT wifi_getApNumDevicesAssociated(INT apIndex, ULONG *output_ulong)
Outputs the number of stations associated per Access Point.
wifi_HS2_Wan_Info_Link_Status_t
UINT rapidReconnThreshold
struct _wifi_key_multi_psk wifi_key_multi_psk_t
INT wifi_getApSecuritySecondaryRadiusServer(INT apIndex, CHAR *IP_output, UINT *Port_output, CHAR *RadiusSecret_output)
Get secondary IP Address, port number and RADIUS server.
INT(* wifi_apDeAuthEvent_callback)(int ap_index, char *mac, int reason)
This call back will be invoked when DeAuth Event (reason 2 wrong password) comes from client.
@ WIFI_MGMT_FRAME_TYPE_REASSOC_RSP
@ WIFI_MGMT_FRAME_TYPE_PROBE_RSP
@ WIFI_ONBOARDINGMETHODS_PHYSICALDISPLAY
INT(* wifi_newApAssociatedDevice_callback)(INT apIndex, wifi_associated_dev_t *associated_dev)
@ wifi_security_mode_wpa3_personal
struct _wifi_associated_dev wifi_associated_dev_t
INT wifi_vapstatus_callback_register(wifi_vapstatus_callback callback)
VAP Status call back registration function.
void(* wifi_receivedAssocReqFrame_callback)(unsigned int ap_index, mac_address_t sta, void *data, unsigned int len)
@ WIFI_ONBOARDINGMETHODS_VIRTUALPUSHBUTTON
INT(* wifi_apDisassociatedDevice_callback)(INT apIndex, char *MAC, INT event_type)
This call back will be invoked when new wifi client disassociates from Access Point.
INT wifi_removeApSecVaribles(INT apIndex)
Deletes internal security variable settings for this access point.
INT wifi_applyGASConfiguration(wifi_GASConfiguration_t *input_struct)
wifi_back_haul_sta_t sta_info
@ WIFI_ONBOARDINGMETHODS_EXTERNALNFCTOKEN
wifi_capabilityListANQP_t capabilityInfo
UINT cli_CapableNumSpatialStreams
INT wifi_setApWpsConfiguration(INT ap_index, wifi_wps_t *wpsConfig)
INT wifi_setApInterworkingServiceEnable(INT apIndex, BOOL input_bool)
Set the Interworking Service enable/disable value for the AP.
INT wifi_getApInterworkingServiceEnable(INT apIndex, BOOL *output_bool)
Get the Interworking Service enable/disable value for the AP.
@ wifi_auth_id_inner_auth_eap
INT wifi_getInterworkingAccessNetworkType(INT apIndex, UINT *output_uint)
UCHAR wifiRoamingConsortiumCount
ULONG wifi_MulticastPacketsSent
INT wifi_getMultiPskClientKey(INT apIndex, mac_address_t mac, wifi_key_multi_psk_t *key)
@ WIFI_ONBOARDINGMETHODS_USBFLASHDRIVE
UINT QueryResponseLengthLimit
INT wifi_getP2PCrossConnect(INT apIndex, BOOL *disabled)
ULONG wifi_DiscardedPacketsReceived
BOOL disable_pmksa_caching
INT wifi_setBroadcastTWTSchedule(INT ap_index, wifi_twt_params_t twtParams, BOOL create, INT *sessionID)
Create or update a broadcast TWT Session
wifi_3gpp_plmn_list_information_element_t plmn_information
@ WIFI_MGMT_FRAME_TYPE_PROBE_REQ
wifi_radius_settings_t radius
@ wifi_ipv6_field_values_not_known
INT wifi_pushMultiPskKeys(INT apIndex, wifi_key_multi_psk_t *keys, INT keysNumber)
@ wifi_security_mode_wep_128
INT wifi_setLayer2TrafficInspectionFiltering(INT apIndex, BOOL enabled)
INT wifi_enableCSIEngine(INT apIndex, mac_address_t sta, BOOL enable)
INT wifi_getApRoamingConsortiumElement(INT apIndex, wifi_roamingConsortiumElement_t *infoElement)
INT wifi_mgmt_frame_callbacks_register(wifi_receivedMgmtFrame_callback mgmtRxCallback)
@ wifi_direction_downlink
INT wifi_setTeardownTWTSession(INT ap_index, INT sessionID)
teardown the individual session or the broadcast session associate to the MAC
INT wifi_addApAclDevice(INT apIndex, CHAR *DeviceMacAddress)
Adds the mac address to the filter list.
INT wifi_setApSecurityRadiusSettings(INT apIndex, wifi_radius_setting_t *input)
Set Access Point security radius settings.
@ wifi_encryption_aes_tkip
@ wifi_security_mode_wpa_enterprise
INT wifi_setApSecurityMFPConfig(INT apIndex, CHAR *MfpConfig)
the hal is used to set the MFP config for each VAP.
@ WIFI_RADIO_SCAN_MODE_SURVEY
INT wifi_getApSecurityRadiusSettings(INT apIndex, wifi_radius_setting_t *output)
Get Access Point security radius settings.
wifi_HS2_NAI_Home_Realm_Query_t realmInfo
@ WIFI_MGMT_FRAME_TYPE_ACTION
INT wifi_kickApAssociatedDevice(INT apIndex, CHAR *client_mac)
Manually removes any active wi-fi association with the device specified on this access point.
wifi_encryption_method_t
Wifi encryption types.
INT wifi_deleteAp(INT apIndex)
Deletes this access point entry on the hardware, clears all internal variables associated with this a...
RADIUS Server information.
INT wifi_setCountryIe(INT apIndex, BOOL enabled)
BOOL bssTransitionActivated
ULONG wifi_UnknownPacketsReceived
wifi_HS2_CapabilityList_t capabilityInfo
This structure hold the information about the wifi interface.
@ wifi_hs2_connection_capability_unknown
ULONG wifi_ErrorsReceived
#define WIFI_AP_MAX_SSID_LEN
wifi_HS2_NAI_Home_Realm_Data_t homeRealmData[20]
@ WIFI_ONBOARDINGMETHODS_EASYCONNECT
wifi_security_key_type_t type
wifi_onboarding_methods_t methodsSupported
int opFriendlyNameInfoLength
INT wifi_getDownStreamGroupAddress(INT apIndex, BOOL *disabled)
wifi_HS2_ConnectionCapability_Status_t
INT wifi_setApSecuritySecondaryRadiusServer(INT apIndex, CHAR *IPAddress, UINT port, CHAR *RadiusSecret)
Set secondary IP Address, port number and RADIUS server, which are used for WLAN security.
ULONG wifi_UnicastPacketsReceived
INT wifi_getLayer2TrafficInspectionFiltering(INT apIndex, BOOL *enabled)
@ WIFI_ONBOARDINGMETHODS_PIN
INT wifi_setApSecurityRadiusServer(INT apIndex, CHAR *IPAddress, UINT port, CHAR *RadiusSecret)
Set the IP Address and port number of the RADIUS server, which are used for WLAN security.
INT wifi_createVAP(wifi_radio_index_t index, wifi_vap_info_map_t *map)
INT wifi_getApWpsConfigurationState(INT apIndex, CHAR *output_string)
Get WPS configuration state.
@ WIFI_ONBOARDINGMETHODS_ETHERNET
@ wifi_security_key_type_pass
wifi_vap_index_t vap_index
wifi_mgmtFrameType_t type
INT wifi_setApSecurity(INT ap_index, wifi_vap_security_t *security)
@ wifi_ipv4_field_values_not_available
wifi_3gppCellularNetwork_t gppInfo
BOOL cli_AuthenticationState
@ wifi_eapol_type_eapol_start
@ WIFI_ONBOARDINGMETHODS_INTEGRATEDNFCTOKEN
INT wifi_setDownStreamGroupAddress(INT apIndex, BOOL disabled)
wifi_security_modes_t securityModesSupported
INT wifi_updateLibHostApdConfig(int apIndex)
INT wifi_getApEnable(INT apIndex, BOOL *output_bool)
Outputs the setting of the internal variable that is set by wifi_setEnable().
wifi_HS2_ConnectionCapability_t connCapabilityInfo
UINT cli_LastDataUplinkRate
INT wifi_sendDataFrame(INT apIndex, mac_address_t sta, UCHAR *data, UINT len, BOOL insert_llc, UINT eth_proto, wifi_data_priority_t prio)
@ wifi_hs2_connection_capability_closed
INT wifi_setApVlanID(INT apIndex, INT vlanId)
Sets the vlan ID for this access point to an internal environment variable.
INT wifi_kickApAclAssociatedDevices(INT apIndex, BOOL enable)
Enable kick for devices on acl black list.
INT wifi_getApAssociatedDevice(INT ap_index, CHAR *output_buf, INT output_buf_size)
Gets the ApAssociatedDevice list for client MAC addresses.
INT wifi_factoryResetAP(int apIndex)
Restore Access point paramters to default without change other AP nor Radio parameters (No need to re...
INT wifi_getApMacAddressControlMode(INT apIndex, INT *output_filterMode)
This function is to read the ACL mode.
UINT eap_identity_req_timeout
@ wifi_security_key_type_psk_sae
@ wifi_security_mode_wpa3_transition
@ wifi_security_mode_wep_64
wifi_vap_security_t security
void wifi_csi_callback_register(wifi_csi_callback callback_proc)
CSI call back registration function. Callback will be executed when the CSI data is available from th...
wifi_security_modes_t mode
@ wifi_ipv4_field_values_not_known
ULONG wifi_BroadcastPacketsSent
@ wifi_security_key_type_sae
@ wifi_hs2_wan_info_link_in_test_state
@ WIFI_MGMT_FRAME_TYPE_AUTH
#define MAX_NUM_VAP_PER_RADIO
INT wifi_getLibhostapd(BOOL *output_bool)
INT wifi_setApWpsButtonPush(INT apIndex)
This function is called when the WPS push button has been pressed for this AP.
@ wifi_ipv4_field_values_port_restricted_single_nated
@ WIFI_ONBOARDINGMETHODS_PHYSICALPUSHBUTTON
@ wifi_security_mode_none
INT wifi_getApName(INT apIndex, CHAR *output_string)
Outputs a 16 byte or less name associated with the Access Point. String buffer must be pre-allocated ...
void(* wifi_sentAssocRspFrame_callback)(unsigned int ap_index, mac_address_t sta, void *data, unsigned int len)
@ WIFI_ONBOARDINGMETHODS_VIRTUALDISPLAY
@ WIFI_MGMT_FRAME_TYPE_ASSOC_REQ
INT wifi_cancelApWPS(INT apIndex)
Cancels WPS mode for this Access Point.
INT wifi_hal_analytics_callback_register(wifi_analytics_callback callback)
VAP Status call back registration function.
wifi_roamingConsortiumElement_t roamingConsortium
void(* wifi_sentAuthFrame_callback)(unsigned int ap_index, mac_address_t sta, void *data, unsigned int len)
This structure hold the information about the wifi interface.
@ wifi_security_mode_wpa_personal
INT wifi_setApMaxAssociatedDevices(INT apIndex, UINT number)
Set maximum associated devices with the Access Point index.
@ WIFI_MGMT_FRAME_TYPE_ASSOC_RSP
INT wifi_setApEnable(INT apIndex, BOOL enable)
Sets the Access Point enable status variable for the specified access point.
CHAR cli_OperatingChannelBandwidth[64]
#define WIFI_AP_MAX_WPSPIN_LEN
INT wifi_getApHotspotElement(INT apIndex, BOOL *enabled)
wifi_radio_index_t radio_index
void(* wifi_receivedAuthFrame_callback)(unsigned int ap_index, mac_address_t sta, void *data, unsigned int len)
#define WIFI_BRIDGE_NAME_LEN
INT wifi_getWifiTrafficStats(INT apIndex, wifi_trafficStats_t *output_struct)
Outputs more detailed traffic stats per AP.
INT wifi_getCountryIe(INT apIndex, BOOL *enabled)
@ WIFI_FRAME_TYPE_INVALID
void wifi_apDeAuthEvent_callback_register(wifi_apDeAuthEvent_callback callback_proc)
Callback registration function.
unsigned int wifi_radio_index_t
INT wifi_setInterworkingAccessNetworkType(INT apIndex, INT accessNetworkType)
UINT blacklist_table_timeout
INT wifi_getApSecurityRadiusServer(INT apIndex, CHAR *IP_output, UINT *Port_output, CHAR *RadiusSecret_output)
Get the IP Address and port number of the RADIUS server, which are used for WLAN security.
wifi_mac_filter_mode_t mac_filter_mode
UINT eap_identity_req_retries
INT wifi_getBssLoad(INT apIndex, BOOL *enabled)
wifi_domainName_t domainNameInfo
wifi_HS2_OperatorFriendlyName_t opFriendlyNameInfo
@ wifi_security_mode_wpa2_personal
wifi_bitrate_t beaconRate
@ wifi_auth_id_credential_type
INT wifi_setApSsidAdvertisementEnable(INT apIndex, BOOL enable)
Sets an internal variable for ssid advertisement.
@ WIFI_ONBOARDINGMETHODS_LABEL
ULONG wifi_UnicastPacketsSent
@ WIFI_EAP_TYPE_NOTIFICATION
@ wifi_security_mode_wpa_wpa2_enterprise
@ wifi_ipv4_field_values_double_nated_private
INT wifi_setApWpsEnrolleePin(INT apIndex, CHAR *pin)
Sets the WPS pin for this Access Point.
UINT identity_req_retry_interval
INT wifi_setApRtsThreshold(INT apIndex, UINT threshold)
Sets the packet size threshold in bytes to apply RTS/CTS backoff rules.
@ WIFI_ONBOARDINGMETHODS_NFCINTERFACE
INT wifi_setP2PCrossConnect(INT apIndex, BOOL disabled)
UINT cli_LastDataDownlinkRate
@ WIFI_RADIO_SCAN_MODE_OFFCHAN
INT(* wifi_receivedMgmtFrame_callback)(INT apIndex, UCHAR *sta_mac, UCHAR *frame, UINT len, wifi_mgmtFrameType_t type, wifi_direction_t dir)
wifi_naiRealmElement_t realmInfo
wifi_security_modes_t
Wifi security mode types.
INT wifi_resetApVlanCfg(INT apIndex)
Reset the vlan configuration for this access point.
ULONG wifi_BroadcastPacketsRecevied
@ wifi_ipv6_field_values_available
INT wifi_setApRetryLimit(INT apIndex, UINT number)
Set the maximum number of retransmission for a packet.
@ wifi_auth_id_expanded_eap
INT wifi_getApRadioIndex(INT apIndex, INT *output_int)
Outputs the radio index for the specified access point.
INT wifi_getApAclDeviceNum(INT apIndex, UINT *output_uint)
Outputs the number of devices in the filter list.
struct _wifi_radius_setting_t wifi_radius_setting_t
RADIUS Server information.
INT RadiusServerRequestTimeout
INT IdentityRequestRetryInterval
INT(* wifi_receivedDataFrame_callback)(INT apIndex, UCHAR *sta_mac, UCHAR *frame, UINT len, wifi_dataFrameType_t type, wifi_direction_t dir)
void(* wifi_received8021xFrame_callback)(unsigned int ap_index, mac_address_t sta, wifi_eapol_type_t type, void *data, unsigned int len)
@ wifi_auth_id_expanded_inner_auth_eap
INT wifi_getTWTsessions(INT ap_index, UINT maxNumberSessions, wifi_twt_sessions_t *twtSessions, UINT *numSessionReturned)
get all the TWT session(individual or Broadcast) connected to that AP Index .
@ wifi_security_key_type_psk
@ wifi_hs2_wan_info_linkdown
wifi_onboarding_methods_t
Wifi onboarding methods.
INT wifi_sendActionFrame(INT apIndex, mac_address_t sta, UINT frequency, UCHAR *frame, UINT len)
INT wifi_setApBeaconRate(INT apIndex, char *sBeaconRate)
Set Access Point Beacon TX rate.
INT wifi_setProxyArp(INT apIndex, BOOL enabled)
@ wifi_ipv6_field_values_not_available
wifi_front_haul_bss_t bss_info
INT wifi_getApWmmEnable(INT apIndex, BOOL *output)
Indicates whether WMM support is currently enabled.
@ wifi_mac_filter_mode_black_list
@ wifi_hs2_wan_info_linkup
@ WIFI_ONBOARDINGMETHODS_DISPLAY
INT wifi_setApSecurityReset(INT apIndex)
When set to true, this AccessPoint instance's WiFi security settings are reset to their factory defau...
@ WIFI_RADIO_SCAN_MODE_FULL
@ wifi_ipv4_field_values_post_restricted
INT wifi_delApAclDevices(INT apINdex)
Get the ACL MAC list per Access Point.
@ WIFI_EAP_TYPE_AKA_PRIME
void(* wifi_sent8021xFrame_callback)(unsigned int ap_index, mac_address_t sta, wifi_eapol_type_t type, void *data, unsigned int len)
wifi_anqp_settings_t anqp
INT wifi_enableGreylistAccessControl(BOOL enable)
This function is to enable or disable grey list Access Control on all applicable VAP.
BOOL BSSTransitionImplemented
wifi_neighborScanMode_t
Represents the wifi scan modes.
wifi_GASConfiguration_t gas_config
INT wifi_setApIsolationEnable(INT apIndex, BOOL enable)
Enables or disables device isolation.
wifi_HS2_WANMetrics_t wanMetricsInfo
@ wifi_eapol_type_eap_packet
wifi_scan_params_t scan_params
INT wifi_setApMacAddressControlMode(INT apIndex, INT filterMode)
Sets the mac address filter control mode.
INT QuietPeriodAfterFailedAuthentication
@ wifi_hs2_connection_capability_open
wifi_roamingConsortium_t roamInfo
void wifi_newApAssociatedDevice_callback_register(wifi_newApAssociatedDevice_callback callback_proc)
Callback registration function.
wifi_venueNameElement_t venueInfo
INT wifi_getProxyArp(INT apIndex, BOOL *enable)
@ wifi_hs2_connection_capability_reserved
@ wifi_ipv4_field_values_available
INT MaxAuthenticationAttempts
INT wifi_getRadioVapInfoMap(wifi_radio_index_t index, wifi_vap_info_map_t *map)
@ WIFI_RADIO_SCAN_MODE_NONE
INT wifi_getApManagementFramePowerControl(INT apIndex, INT *output_dBm)
Get the ApManagementFramePowerControl.
INT BlacklistTableTimeout
@ wifi_eapol_type_eapol_key
CHAR cli_InterferenceSources[64]
@ WIFI_DATA_FRAME_TYPE_INVALID
@ WIFI_ONBOARDINGMETHODS_PUSHBUTTON
@ wifi_mac_filter_mode_white_list
@ WIFI_RADIO_SCAN_MODE_ONCHAN
INT wifi_setApDASRadiusServer(INT apIndex, CHAR *IPAddress, UINT port, CHAR *RadiusdasSecret)
Set the IP Address and port number of the RADIUS DAS server, which are used for WLAN security.
@ wifi_hs2_wan_info_reserved
@ WIFI_DATA_FRAME_TYPE_8021x
INT wifi_getMultiPskKeys(INT apIndex, wifi_key_multi_psk_t *keys, INT keysNumber)
INT wifi_pushApHotspotElement(INT apIndex, BOOL enabled)
BOOL network_initiated_greylist
INT wifi_getApStatus(INT apIndex, CHAR *output_string)
Outputs the AP "Enabled" "Disabled" status from driver.
@ wifi_security_mode_wpa2_enterprise
@ wifi_security_mode_wpa3_enterprise
BOOL wpa3_transition_disable
BOOL rtsThresholdSupported
INT wifi_getAPCapabilities(INT ap_index, wifi_ap_capabilities_t *apCapabilities)
wifi_interworking_t interworking
@ wifi_security_mode_wpa_wpa2_personal
INT wifi_getApBeaconRate(INT apIndex, char *output_BeaconRate)
Get Access Point Beacon TX rate.
INT wifi_setApManagementFramePowerControl(INT apIndex, INT dBm)
Sets the ApManagementFramePowerControl.
INT wifi_getApSecurity(INT ap_index, wifi_vap_security_t *security)
unsigned char mac_address_t[6]
ULONG wifi_DiscardedPacketsSent
UINT cli_AuthenticationFailures
INT(* wifi_analytics_callback)(CHAR *fmt,...)
This call back will be invoked when HAL wants to log catasrophic failures.
CHAR cli_OperatingStandard[64]
struct _wifi_trafficStats wifi_trafficStats_t
wifi_vapstatus_t
VAP status possible values.
BOOL interworkingServiceSupported
wifi_encryption_method_t encr
INT wifi_getApRetryLimit(INT apIndex, UINT *output)
Get the maximum number of retransmission for a packet.
@ WIFI_MGMT_FRAME_TYPE_DEAUTH
wifi_InterworkingElement_t interworking
INT wifi_getApInterworkingElement(INT apIndex, wifi_InterworkingElement_t *output_struct)
Get the Interworking Element that will be sent by the AP.
ULONG cli_DataFramesSentAck
INT wifi_getApSecurityMFPConfig(INT apIndex, CHAR *output_string)
To retrive the MFPConfig for each VAP.
@ wifi_ipv4_field_values_port_restricted_double_nated
@ WIFI_MGMT_FRAME_TYPE_INVALID
wifi_connection_status_t conn_status
@ wifi_ipv4_field_values_single_nated_private
@ WIFI_MGMT_FRAME_TYPE_REASSOC_REQ
INT wifi_disableApEncryption(INT apIndex)
Changes the hardware settings to disable encryption on this access point.
INT wifi_setApWmmEnable(INT apIndex, BOOL enable)
Enables/disables WMM on the hardwawre for this AP. enable==1, disable == 0.
@ wifi_auth_id_tunneled_eap