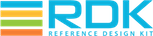 |
RDK-B
|
Go to the documentation of this file.
19 #ifndef __WIFI_HAL_RADIO_H__
20 #define __WIFI_HAL_RADIO_H__
42 #define MAXNUMSECONDARYCHANNELS 7
280 #ifdef WIFI_HAL_VERSION_3_PHASE2
wifi_ieee80211Variant_t
Wifi 802.11 variant Types.
INT wifi_getRadioCarrierSenseThresholdRange(INT radioIndex, INT *output)
Indicates the Carrier Sense ranges supported by the radio.
INT wifi_getGuardInterval(INT radio_index, wifi_guard_interval_t *guard_interval)
INT wifi_setBSSColor(INT radio_index, UCHAR color)
@ wifi_dl_data_block_ack_immediate
wifi_channelState_t ch_state
Enhanced Distributed Channel Access parameters.
@ wifi_guard_interval_400
@ wifi_guard_interval_800
INT wifi_setRadioDfsAtBootUpEnable(INT radioIndex, BOOL enable)
Set the Dfs enable on Bootup status.
INT wifi_getRadioResetCount(INT radioIndex, ULONG *output_int)
Get the radio reset count.
@ WIFI_EVENT_RADAR_PRE_CAC_EXPIRED
@ WIFI_EVENT_RADAR_NOP_FINISHED
INT wifi_getRadioAutoBlockAckEnable(INT radioIndex, BOOL *output_bool)
Get radio auto block ack enable setting.
wifi_guard_interval_t guardInterval
INT wifi_getRadioIGMPSnoopingEnable(INT radioIndex, BOOL *output_bool)
Get radio IGMP snooping enable setting.
INT wifi_getRadioDfsAtBootUpEnable(INT radioIndex, BOOL *enable)
Get the Dfs enable on Bootup status.
INT wifi_getBSSColor(INT radio_index, UCHAR *color)
UINT operationalDataTransmitRates
@ CHAN_STATE_DFS_NOP_START
INT wifi_setRadioMCS(INT radioIndex, INT MCS)
Set the Modulation Coding Scheme index, eg: "-1", "1", "15".
int wifi_factoryResetRadios()
Restore all radio parameters without touching access point parameters.
INT wifi_setRadioTransmitPower(INT radioIndex, ULONG TransmitPower)
Set current Transmit Power, eg "75", "100".
INT wifi_setRadioAMSDUEnable(INT radioIndex, BOOL amsduEnable)
Enables A-MSDU in the hardware, 0 == not enabled, 1 == enabled.
INT wifi_getRadioStatus(INT radioIndex, BOOL *output_bool)
Get the Radio enable status.
#define MAXNUMSECONDARYCHANNELS
INT wifi_getRadioPercentageTransmitPower(INT radioIndex, ULONG *output_ulong)
Get current Transmit Power level in units of full power.
INT wifi_getRadioUpTime(INT radioIndex, ULONG *uptime)
Get the number of seconds elapsed since radio is started.
INT wifi_getRadioReverseDirectionGrantSupported(INT radioIndex, BOOL *output_bool)
Get radio RDG enable Support.
wifi_freq_bands_t
Wifi Frequency Band Types.
INT wifi_setUplinkMuType(INT radio_index, wifi_ul_mu_type_t mu_type)
INT wifi_setRadioSTBCEnable(INT radioIndex, BOOL STBC_Enable)
Enable STBC mode in the hardware. 0 == not enabled, 1 == enabled.
wifi_channelBandwidth_t
Wifi Channel Bandwidth Types.
UINT radioStatsMeasuringRate
UINT fragmentationThreshold
INT wifi_getRadioAMSDUEnable(INT radioIndex, BOOL *output_bool)
Outputs A-MSDU enable status, 0 == not enabled, 1 == enabled.
INT wifi_applyRadioSettings(INT radioIndex)
This API is used to apply (push) all previously set radio level variables and make these settings act...
INT wifi_getRadioMCS(INT radioIndex, INT *output_INT)
Get the Modulation Coding Scheme index, eg: "-1", "1", "15".
INT wifi_getUplinkMuType(INT radio_index, wifi_ul_mu_type_t *mu_type)
wifi_guard_interval_t
Guard intervals types.
INT wifi_getDownlinkMuType(INT radio_index, wifi_dl_mu_type_t *mu_type)
@ wifi_dl_data_ack_immediate
@ WIFI_EVENT_RADAR_CAC_STARTED
INT wifi_getRadioChannelsInUse(INT radioIndex, CHAR *output_string)
Get the list of supported channel. eg: "1-11".
INT wifi_setRadioFragmentationThreshold(INT apIndex, UINT threshold)
Sets the fragmentation threshold in bytes for the radio used by this Access Point.
void wifi_scanResults_callback_register(wifi_scanResults_callback callback_proc)
@ wifi_guard_interval_auto
unsigned int wifi_radio_index_t
INT wifi_setDownlinkDataAckType(INT radio_index, wifi_dl_data_ack_type_t ack_type)
INT wifi_setRadioObssCoexistenceEnable(INT apIndex, BOOL enable)
Enables OBSS Coexistence - fall back to 20MHz if necessary for the radio used by this AP.
INT wifi_getRadioIfName(INT radioIndex, CHAR *output_string)
Get the Radio Interface name from platform, eg "wifi0".
@ WIFI_EVENT_RADAR_DETECTED
INT wifi_setRadioDfsEnable(INT radioIndex, BOOL enabled)
Set the Dfs enable status.
INT wifi_getRadioCarrierSenseThresholdInUse(INT radioIndex, INT *output)
The RSSI signal level at which CS/CCA detects a busy condition.
INT wifi_setDownlinkMuType(INT radio_index, wifi_dl_mu_type_t mu_type)
INT wifi_getRadioDfsEnable(INT radioIndex, BOOL *output_bool)
Get the Dfs enable status.
@ WIFI_EVENT_RADAR_CAC_FINISHED
BOOL chanUtilSelfHealEnable
INT wifi_getAvailableBSSColor(INT radio_index, INT maxNumberColors, UCHAR *colorList, INT *numColorReturned)
Get the list of avaiable BSS color.
INT wifi_setRadioCtsProtectionEnable(INT apIndex, BOOL enable)
Enables CTS protection for the radio used by this Access Point.
INT wifi_getRadioOperatingParameters(wifi_radio_index_t index, wifi_radio_operationParam_t *operationParam)
Get Radio Operating Parameters.
struct _wifi_channelMap_t wifi_channelMap_t
@ wifi_dl_data_block_ack_deferred
UINT radioStatsMeasuringInterval
INT(* wifi_scanResults_callback)(wifi_radio_index_t index, wifi_bss_info_t **bss, UINT *num_bss)
wifi_access_category_t
Wifi access category (AC) type.
INT wifi_getRadioTransmitPower(INT radioIndex, ULONG *output_ulong)
Get current Transmit Power in dBm units.
INT wifi_setRadioEnable(INT radioIndex, BOOL enable)
Set the Radio enable config parameter.
INT wifi_setRadioOperatingParameters(wifi_radio_index_t index, wifi_radio_operationParam_t *operationParam)
Set Radio Operating Parameters.
INT wifi_getScanResults(wifi_radio_index_t index, wifi_channel_t *channel, wifi_bss_info_t **bss, UINT *num_bss)
wifi_operating_env_t operatingEnvironment
INT wifi_setGuardInterval(INT radio_index, wifi_guard_interval_t guard_interval)
INT wifi_getMuEdca(INT radio_index, wifi_access_category_t ac, wifi_edca_t *edca)
Get MU (Multi-User) EDCA (Enhanced Distributed Channel Access) parameter.
wifi_ieee80211Variant_t variant
wifi_countrycode_type_t countryCode
INT wifi_getZeroDFSState(UINT radioIndex, BOOL *enable, BOOL *precac)
Get Zero DFS State.
INT wifi_setRadioAutoBlockAckEnable(INT radioIndex, BOOL enable)
Set radio auto block ack enable setting.
@ CHAN_STATE_DFS_CAC_COMPLETED
INT wifi_setRadioIGMPSnoopingEnable(INT radioIndex, BOOL enable)
Set radio IGMP snooping enable setting.
@ wifi_guard_interval_3200
@ wifi_guard_interval_1600
INT wifi_setRadioCarrierSenseThresholdInUse(INT radioIndex, INT threshold)
Set Carrier sense threshold in use for the selected radio index.
UINT basicDataTransmitRates
@ WIFI_EVENT_RADAR_CAC_ABORTED
INT wifi_get80211axDefaultParameters(INT radio_index, wifi_80211ax_params_t *params)
@ CHAN_STATE_DFS_CAC_START
int wifi_factoryResetRadio(int radioIndex)
Restore selected radio parameters without touching access point parameters.
UINT numSecondaryChannels
INT wifi_setZeroDFSState(UINT radioIndex, BOOL enable, BOOL precac)
Set Zero DFS State.
@ CHAN_STATE_DFS_NOP_FINISHED
INT wifi_getRadioEnable(INT radioIndex, BOOL *output_bool)
Get the Radio enable config parameter.