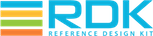 |
RDK-B
|
Go to the documentation of this file.
19 #ifndef __WIFI_HAL_TELEMETRY_H__
20 #define __WIFI_HAL_TELEMETRY_H__
141 #ifdef WIFI_HAL_VERSION_3_PHASE2
156 #define MAX_VAP (MAX_NUM_RADIOS*MAX_NUM_VAP_PER_RADIO)
213 #ifdef WIFI_HAL_VERSION_3_PHASE2
258 #ifdef WIFI_HAL_VERSION_3_PHASE2
ULONG ssid_MultipleRetryCount
INT wifi_getRadioBandUtilization(INT radioIndex, INT *output_percentage)
To read the radio band utilization.
INT wifi_setRadioStatsEnable(INT radioIndex, BOOL enable)
This API is to enable/disable radio status.
ULONG ssid_MulticastPacketsSent
char ap_OperatingFrequencyBand[16]
struct _wifi_ssidTrafficStats2 wifi_ssidTrafficStats2_t
ULONG ssid_MulticastPacketsReceived
ULONG ssid_DiscardedPacketsReceived
INT radio_MedianNoiseFloorOnChannel
ULONG ssid_AggregatedPacketCount
char ap_SecurityModeEnabled[64]
struct _wifi_rssi_snapshot wifi_rssi_snapshot_t
char ap_EncryptionMode[64]
INT radio_MaximumNoiseFloorOnChannel
UINT ap_ChannelUtilization
ULONG ssid_DiscardedPacketsSent
ULONG ssid_ACKFailureCount
ULONG radio_PacketsReceived
ULONG ssid_UnicastPacketsSent
wifi_channelBandwidth_t
Wifi Channel Bandwidth Types.
char ap_OperatingChannelBandwidth[16]
ULONG ssid_BroadcastPacketsSent
char ap_BasicDataTransferRates[256]
INT wifi_getRadioStatsEnable(INT radioIndex, BOOL *output_enable)
This API returns the radio enabled status.
INT wifi_getApAssociatedDeviceDiagnosticResult3(INT apIndex, wifi_associated_dev3_t **associated_dev_array, UINT *output_array_size)
INT wifi_setClientDetailedStatisticsEnable(INT radioIndex, BOOL enable)
This function enabled/disabled collection of detailed statistics of associated clients on Access Poin...
ULONG ssid_FailedRetransCount
This structure hold the information about the wifi interface.
ULONG radio_ErrorsReceived
ULONG radio_PLCPErrorCount
ULONG radio_PacketsOtherReceived
ULONG radio_StatisticsStartTime
INT wifi_getRadioTrafficStats2(INT radioIndex, wifi_radioTrafficStats2_t *output_struct)
Get detail radio traffic static info.
char ap_SupportedDataTransferRates[256]
INT radio_MinimumNoiseFloorOnChannel
ULONG radio_DiscardPacketsSent
struct _wifi_neighbor_ap2 wifi_neighbor_ap2_t
INT radio_RetransmissionMetirc
ULONG radio_ChannelUtilization
char ap_OperatingStandards[16]
INT wifi_getApAssociatedClientDiagnosticResult(INT apIndex, char *mac_addr, wifi_associated_dev3_t *dev_conn)
struct _wifi_apRssi wifi_apRssi_t
ULONG radio_BytesReceived
INT wifi_getNeighboringWiFiStatus(INT radioIndex, wifi_neighbor_ap2_t **neighbor_ap_array, UINT *output_array_size)
Returns the Wifi scan status.
INT radio_CarrierSenseThreshold_Exceeded
ULONG radio_InvalidMACCount
ULONG ssid_UnknownPacketsReceived
ULONG ssid_BroadcastPacketsRecevied
struct _wifi_radioTrafficStats2 wifi_radioTrafficStats2_t
INT wifi_getVAPTelemetry(UINT apIndex, wifi_VAPTelemetry_t *telemetry)
char ap_SupportedStandards[64]
ULONG radio_DiscardPacketsReceived
VAP Telemetry information.
ULONG radio_FCSErrorCount
unsigned char mac_address_t[6]
ULONG ssid_ErrorsReceived
ULONG ssid_UnicastPacketsReceived
INT wifi_getSSIDTrafficStats2(INT ssidIndex, wifi_ssidTrafficStats2_t *output_struct)
Get the basic SSID traffic static info.
ULONG ssid_PacketsReceived