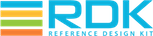 |
RDK-B
|
Go to the documentation of this file.
20 #ifndef __WIFI_HAL_EXTENDER_H__
21 #define __WIFI_HAL_EXTENDER_H__
291 #ifdef WIFI_HAL_VERSION_3_PHASE2
406 #define MAX_BTM_DEVICES 64
407 #define MAX_URL_LEN 512
408 #define MAX_CANDIDATES 64
409 #define MAX_VENDOR_SPECIFIC 32
616 #define MAX_REQUESTED_ELEMS 8
617 #define MAX_CHANNELS_REPORT 16
920 #ifdef WIFI_HAL_VERSION_3_PHASE2
1040 UINT steeringgroupIndex,
1058 UINT steeringgroupIndex,
1082 UINT steeringgroupIndex,
1101 UINT steeringgroupIndex,
1114 #ifdef WIFI_HAL_VERSION_3_PHASE2
1254 #ifdef WIFI_HAL_VERSION_3_PHASE2
1305 UINT *out_array_size,
1306 UCHAR *out_DialogToken);
1348 #ifdef WIFI_HAL_VERSION_3_PHASE2
1369 UCHAR *out_DialogToken);
Configuration per apIndex.
UINT inactCheckThresholdSec
INT wifi_setRMBeaconRequest(UINT apIndex, CHAR *peer, wifi_BeaconRequest_t *in_request, UCHAR *out_DialogToken)
BOOL requestedElementIDSPresent
wifi_VendorSpecific_t vendorSpecific
@ post_assoc_active_kickmac
wifi_steering_rssiChange_t inactveXing
BOOL condensedCountrySringPresent
#define MAX_VENDOR_SPECIFIC
@ WIFI_STEERING_RSSI_UNCHANGED
UINT utilCheckIntervalSec
wifi_rssi_snapshot_t cli_rssi_ack
Client Activity Change Event Data This data is provided with WIFI_STEERING_EVENT_CLIENT_ACTIVITY.
unsigned int wifi_steer_matching_condition_t
STA datarate information These are STA capabilities values.
wifi_steering_datarateInfo_t datarateInfo
wifi_steering_rssiChange_t
RSSI Crossing Values These are the RSSI crossing values provided in RSSI crossing events.
wifi_steering_rrmCaps_t rrmCaps
INT wifi_steering_clientSet(UINT steeringgroupIndex, INT apIndex, mac_address_t client_mac, wifi_steering_clientConfig_t *config)
Add Client Config to apIndex.
INT wifi_setApScanFilter(INT apIndex, INT mode, CHAR *essid)
Enable/Disable scan filter in the driver.
@ post_assoc_idle_kick_mac
wifi_CondensedCountryString_t condensedCountryStr
@ DISCONNECT_SOURCE_REMOTE
Configuration per Client.
wifi_WideBWChannel_t wideBandwidthChannel
@ WIFI_STEERING_EVENT_CHAN_UTILIZATION
struct _wifi_associated_dev_rate_info_tx_stats wifi_associated_dev_rate_info_tx_stats_t
wifi_VHTCapabilities_t vbhtCaps
@ WIFI_STEERING_EVENT_AUTH_FAIL
INT wifi_getApAssociatedDeviceTidStatsResult(INT radioIndex, mac_address_t *clientMacAddress, wifi_associated_dev_tid_stats_t *tid_stats, ULLONG *handle)
Get the associated client per rate transmission status.
wifi_HTCapabilities_t htCaps
wifi_VHTOperation_t vhtOp
void(* wifi_steering_eventCB_t)(UINT steeringgroupIndex, wifi_steering_event_t *event)
Wifi Steering Event Callback Definition.
Probe Request Event Data This data is provided with WIFI_STEERING_EVENT_PROBE_REQ.
USHORT randomizationInterval
wifi_WideBWChannel_t opInfo
wifi_ChannelReport_t channelReport
struct wifi_associated_dev_tid_stats wifi_associated_dev_tid_stats_t
INT wifi_steering_setGroup(UINT steeringgroupIndex, wifi_steering_apConfig_t *cfg_2, wifi_steering_apConfig_t *cfg_5)
Add a Steering Group.
@ DISCONNECT_TYPE_UNKNOWN
wifi_RequestedElementIDS_t wifi_ExtdRequestedElementIDS_t
INT wifi_getRMCapabilities(CHAR *peer, UCHAR out_Capabilities[5])
wifi_steering_evConnect_t connect
BOOL vendorSpecificPresent
struct _wifi_associated_dev_stats wifi_associated_dev_stats_t
BOOL beaconReportingPresent
@ WIFI_STEERING_RSSI_LOWER
struct _wifi_channelStats wifi_channelStats_t
INT wifi_steering_eventUnregister(void)
Unregister for Steering Event Callbacks.
BOOL rmEnabledCapsPresent
Auth Failure Event Data This data is provided with WIFI_STEERING_EVENT_AUTH_FAIL.
wifi_BSSTransitionCandidatePreference_t bssTransitionCandidatePreference
wifi_freq_bands_t
Wifi Frequency Band Types.
INT wifi_getApAssociatedDeviceTxStatsResult(INT radioIndex, mac_address_t *clientMacAddress, wifi_associated_dev_rate_info_tx_stats_t **stats_array, UINT *output_array_size, ULLONG *handle)
Get the associated client per rate transmission status.
BOOL channelReportPresent
wifi_steering_rssiChange_t highXing
wifi_disconnectSource_t
Wifi Disconnect Sources.
wifi_associated_dev_tid_entry_t tid_array[16]
struct _wifi_associated_dev_rate_info_rx_stats wifi_associated_dev_rate_info_rx_stats_t
wifi_RequestedElementIDS_t requestedElementIDS
wifi_ExtdRequestedElementIDS_t extdRequestedElementIDS
INT wifi_steering_eventRegister(wifi_steering_eventCB_t event_cb)
Register for Steering Event Callbacks.
Client Disconnect Event Data This data is provided with WIFI_STEERING_EVENT_CLIENT_DISCONNECT.
BOOL bssTransitionCandidatePreferencePresent
@ WIFI_STEERING_RSSI_HIGHER
BOOL extdRequestedElementIDSPresent
void wifi_steerTriggered_callback_register(wifi_steerTriggered_callback callback_proc, CHAR *module)
wifi_WideBWChannel_t wideBandwidthChannel
wifi_disconnectSource_t source
wifi_BTMTerminationDuration_t btmTerminationDuration
wifi_rssi_snapshot_t cli_rssi_bcn
BOOL btmTerminationDurationPresent
INT wifi_cancelRMBeaconRequest(UINT apIndex, UCHAR dialogToken)
wifi_WideBWChannel_t wideBandwidthChannel
@ WIFI_STEERING_EVENT_CLIENT_CONNECT
@ WIFI_STEERING_EVENT_RSSI_XING
Client Connect Event Data This data is provided with WIFI_STEERING_EVENT_CLIENT_CONNECT.
BOOL reportingRetailPresent
wifi_steering_evDisconnect_t disconnect
INT wifi_applySSIDSettings(INT ssidIndex)
Apply SSID and AP (in the case of Acess Point devices) to the hardware.
USHORT rxHighestSupportedRate
INT(* wifi_steerTriggered_callback)(INT apIndex, wifi_steer_trigger_data_t *data)
ULLONG ch_utilization_busy
INT(* wifi_BTMQueryRequest_callback)(UINT apIndex, CHAR *peerMac, wifi_BTMQuery_t *query, UINT inMemSize, wifi_BTMRequest_t *request)
This call back is invoked when a STA sends a BTM query message to a vAP in the gateway....
wifi_steer_matching_condition_t cond
wifi_RMEnabledCapabilities_t rmEnabledCaps
INT wifi_getApAssociatedDeviceStats(INT apIndex, mac_address_t *clientMacAddress, wifi_associated_dev_stats_t *associated_dev_stats, ULLONG *handle)
Get the associated device status.
INT wifi_steering_clientRemove(UINT steeringgroupIndex, INT apIndex, mac_address_t client_mac)
Remove Client Config from apIndex.
@ WIFI_STEERING_EVENT_CLIENT_ACTIVITY
wifi_disconnectType_t
Wifi Disconnect Types These are the types of wifi disconnects.
@ DISCONNECT_SOURCE_UNKNOWN
wifi_steering_evActivity_t activity
Channel Utilization Event Data This data is provided with WIFI_STEERING_EVENT_CHAN_UTILIZATION.
wifi_steering_evProbeReq_t probeReq
INT wifi_steering_clientDisconnect(UINT steeringgroupIndex, INT apIndex, mac_address_t client_mac, wifi_disconnectType_t type, UINT reason)
Initiate a Client Disconnect.
BOOL wideBandWidthChannelPresent
wifi_SecondaryChannelOffset_t secondaryChannelOffset
@ DISCONNECT_SOURCE_LOCAL
Wifi Steering Event This is the data containing a single steering event.
ULLONG ch_utilization_busy_tx
BOOL vendorSpecificPresent
INT(* wifi_RMBeaconReport_callback)(UINT apIndex, wifi_BeaconReport_t *out_struct, UINT *out_array_size, UCHAR *out_DialogToken)
INT(* wifi_BTMResponse_callback)(UINT apIndex, CHAR *peerMac, wifi_BTMResponse_t *response)
This call back is invoked when a STA responds to a BTM Request from the gateway.
wifi_disconnectType_t type
INT wifi_setBTMRequest(UINT apIndex, CHAR *peerMac, wifi_BTMRequest_t *request)
Set a BTM Request to a non-AP STA. The callback register function should be called first so that the ...
INT wifi_startNeighborScan(INT apIndex, wifi_neighborScanMode_t scan_mode, INT dwell_time, UINT chan_num, UINT *chan_list)
This API initates the scanning.
USHORT txHighestSupportedRate
@ DISCONNECT_TYPE_DISASSOC
@ WIFI_STEERING_EVENT_PROBE_REQ
wifi_neighborScanMode_t
Represents the wifi scan modes.
#define MAX_REQUESTED_ELEMS
wifi_MeasurementPilotTransmission_t msmtPilotTransmission
BOOL wideBandWidthChannelPresent
wifi_steering_evAuthFail_t authFail
INT wifi_BTMQueryRequest_callback_register(UINT apIndex, wifi_BTMQueryRequest_callback btmQueryCallback, wifi_BTMResponse_callback btmResponseCallback)
BTM Query callback registration function.
INT wifi_steering_clientMeasure(UINT steeringgroupIndex, INT apIndex, mac_address_t client_mac)
Initiate Instant Client RSSI Measurement.
ULLONG ch_utilization_busy_rx
@ WIFI_STEERING_EVENT_CLIENT_DISCONNECT
wifi_steering_eventType_t
Wifi Steering Event Types These are the different steering event types that are sent by the wifi_hal ...
INT wifi_RMBeaconRequestCallbackUnregister(UINT apIndex, wifi_RMBeaconReport_callback beaconReportCallback)
wifi_steering_rssiChange_t lowXing
INT wifi_setApCsaDeauth(INT apIndex, INT mode)
This API set the CSA (Channel Switch Announcement) deauthentication to all clients before moving to a...
wifi_steering_evRssiXing_t rssiXing
wifi_steering_eventType_t type
BOOL msmtPilotTransmissionPresent
wifi_steering_evChanUtil_t chanUtil
BOOL secondaryChannelOffsetPresent
wifi_BTMTerminationDuration_t termDuration
INT wifi_RMBeaconRequestCallbackRegister(UINT apIndex, wifi_RMBeaconReport_callback beaconReportCallback)
INT wifi_getRadioChannelStats(INT radioIndex, wifi_channelStats_t *input_output_channelStats_array, INT array_size)
Get the channels utilization status.
INT wifi_getSSIDRadioIndex(INT ssidIndex, INT *radioIndex)
Get the radio index associated with the SSID entry.
wifi_BeaconReporting_t beaconReporting
wifi_steering_evRssi_t rssi
struct wifi_associated_dev_tid_entry wifi_associated_dev_tid_entry_t
ULLONG ch_utilization_busy_self
wifi_VendorSpecific_t vendorSpecific
BOOL wideBandWidthChannelPresent
#define MAX_CHANNELS_REPORT
ULLONG ch_utilization_total
UCHAR disassociationImminent
unsigned char mac_address_t[6]
@ WIFI_STEERING_EVENT_RSSI
ULLONG ch_utilization_busy_ext
@ post_assoc_active_80211v
UINT inactCheckIntervalSec
wifi_VendorSpecific_t vendorSpecific
INT wifi_getApAssociatedDeviceRxStatsResult(INT radioIndex, mac_address_t *clientMacAddress, wifi_associated_dev_rate_info_rx_stats_t **stats_array, UINT *output_array_size, ULLONG *handle)
Get the associated client per rate receive status.